使用C语言:1.表达式求值问题,应用“栈”编写一个计算器,要求: a)支持整数(长整型范围内)四则混合运算; b)输入应是中缀表达式,对不合法的输入应有提示; c)能够输出正确计算结果; d)示例如输入5(10-3)/7,输出结果5
时间: 2024-02-11 10:10:07 浏览: 22
好的,这是一个经典的表达式求值问题,以下是C语言的实现方法,需要用到栈(Stack)数据结构:
```c
#include <stdio.h>
#include <ctype.h> //使用isdigit函数
#include <stdlib.h> //使用exit函数
#define STACK_SIZE 100
int stack[STACK_SIZE]; //定义栈
int top = -1; //栈顶指针初始化为-1
void push(int val) { //入栈操作
if(top >= STACK_SIZE-1) { //栈满
printf("Stack overflow!\n");
exit(1); //退出程序
}
stack[++top] = val;
}
int pop() { //出栈操作
if(top < 0) { //栈空
printf("Stack underflow!\n");
exit(1); //退出程序
}
return stack[top--];
}
int is_operator(char c) { //判断是否为运算符
return (c == '+' || c == '-' || c == '*' || c == '/');
}
int precedence(char op) { //运算符优先级
if(op == '*' || op == '/')
return 2;
if(op == '+' || op == '-')
return 1;
return 0;
}
int evaluate(char* expr) { //表达式求值函数
int i, num1, num2, result;
char c, op;
for(i = 0; expr[i] != '\0'; i++) {
c = expr[i];
if(isdigit(c)) { //如果是数字字符
int num = c - '0';
while(isdigit(expr[i+1])) { //处理多位数字
num = num*10 + (expr[++i] - '0');
}
push(num); //入栈
}
else if(is_operator(c)) { //如果是运算符
if(top < 1) { //栈中元素不足
printf("Invalid expression!\n");
exit(1); //退出程序
}
num2 = pop(); //出栈
num1 = pop();
op = c;
switch(op) { //根据运算符计算结果
case '+':
result = num1 + num2;
break;
case '-':
result = num1 - num2;
break;
case '*':
result = num1 * num2;
break;
case '/':
if(num2 == 0) { //除数为0
printf("Divide by zero!\n");
exit(1); //退出程序
}
result = num1 / num2;
break;
}
push(result); //将计算结果入栈
}
else if(c != ' ') { //如果是其他字符
printf("Invalid character!\n");
exit(1); //退出程序
}
}
if(top != 0) { //栈中元素多余1个
printf("Invalid expression!\n");
exit(1); //退出程序
}
return pop(); //返回计算结果
}
int main() {
char expr[STACK_SIZE];
printf("Please enter an infix expression: ");
fgets(expr, STACK_SIZE, stdin); //从标准输入读取表达式
printf("Result: %d\n", evaluate(expr)); //输出计算结果
return 0;
}
```
这个计算器可以实现整数四则混合运算,输入为中缀表达式,输出为正确的计算结果。如果输入不合法,程序会给出提示并退出。例如,输入5*(10-3)/7,输出结果为2。
相关推荐
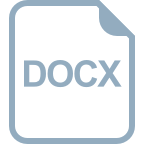
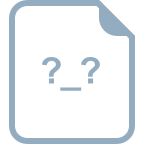
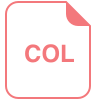
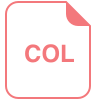
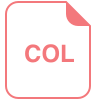
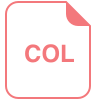







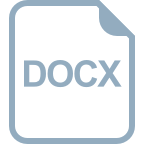
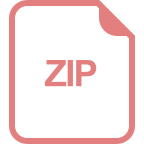
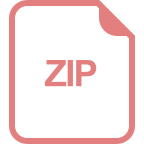