下面代码中的user是什么?在IDEA环境下,如何创造这样一个“user”? 代码:<?xml version="1.0" encoding="UTF-8" ?><!DOCTYPE mapperPUBLIC "-//mybatis.org//DTD Mapper 3.0//EN""http://mybatis.org/dtd/mybatis-3-mapper.dtd"><mapper namespace="com.example.demo.mapper.UserMapper"> <resultMap id="userMap" type="com.example.demo.entity.User"> <id column="id" property="id"/> <result column="name" property="name"/> <result column="age" property="age"/> <result column="gender" property="gender"/> </resultMap> <select id="selectById" resultMap="userMap"> select * from user where id = #{id} </select> <select id="selectAll" resultMap="userMap"> select * from user </select> <insert id="insert" parameterType="com.example.demo.entity.User"> insert into user(name, age, gender) values(#{name}, #{age}, #{gender}) </insert> <update id="update" parameterType="com.example.demo.entity.User"> update user set name = #{name}, age = #{age}, gender = #{gender} where id = #{id} </update> <delete id="deleteById"> delete from user where id = #{id} </delete></mapper>
时间: 2023-12-05 17:47:34 浏览: 34
在这个XML文件中,"user"是一个数据库表的实体类,包含了id、name、age、gender等字段。这里的"user"并不是IDEA环境下创建的对象,而是代表了数据库表的实体类,需要在Java代码中创建对应的实体类。
在IDEA环境下创建一个类,可以在Project视图中右键点击src目录,选择New->Java Class,然后设置类名为"User",并添加相应的属性(id、name、age、gender)。在这个类中,需要添加对应的getter和setter方法以及构造函数,以便在MyBatis映射中使用。
相关问题
如何java.sql.SQLException: Access denied for user 'root'@'localhost' (using password: YES)在idea的maven lib中添加一个mysql-connector
要在IDEA的Maven项目中添加MySQL连接器,你需要在项目的pom.xml文件中添加以下依赖项:
```xml
<dependencies>
<!-- 其他依赖项 -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.26</version>
</dependency>
</dependencies>
```
请确保将`<version>`元素设置为您正在使用的MySQL服务器版本的相应版本号。然后保存pom.xml文件,Maven将自动下载并添加MySQL连接器到项目的构建路径中。
如果你已经添加了这个依赖项但仍然遇到`java.sql.SQLException: Access denied for user 'root'@'localhost' (using password: YES)`错误,可能是由于数据库连接配置的问题导致的。请确保在连接字符串中指定正确的用户名、密码和数据库名称,并确保MySQL服务器正在运行并接受来自本地主机的连接。
希望这可以帮助你解决问题!如有任何进一步的疑问,请随时提问。
idea写一个javaweb实现登录注册基于(spring mvc,mysql,maven)的代码并且将项目完整结构写下来
好的,以下是一个基于 Spring MVC、MySQL 和 Maven 的简单登录注册系统的代码示例及其完整项目结构:
1. 项目结构
```
|- src/main/java
|- com.example.demo
|- controller
|- UserController.java
|- dao
|- UserDAO.java
|- model
|- User.java
|- service
|- UserService.java
|- UserServiceImpl.java
|- src/main/resources
|- application.properties
|- log4j.properties
|- src/main/webapp
|- WEB-INF
|- views
|- index.jsp
|- login.jsp
|- register.jsp
|- web.xml
|- index.jsp
|- login.jsp
|- register.jsp
|- pom.xml
```
2. 相关依赖和配置
pom.xml:
```xml
<dependencies>
<!-- Spring MVC -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>5.3.6</version>
</dependency>
<!-- MySQL -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.23</version>
</dependency>
<!-- MyBatis -->
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>3.5.7</version>
</dependency>
<!-- MyBatis-Spring -->
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis-spring</artifactId>
<version>2.0.6</version>
</dependency>
<!-- Spring JDBC -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>5.3.6</version>
</dependency>
<!-- Spring TX -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-tx</artifactId>
<version>5.3.6</version>
</dependency>
<!-- Spring Test -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-test</artifactId>
<version>5.3.6</version>
</dependency>
<!-- Junit -->
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
<scope>test</scope>
</dependency>
</dependencies>
```
application.properties:
```properties
# MySQL 数据库配置
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
spring.datasource.url=jdbc:mysql://localhost:3306/user_db?useSSL=false&serverTimezone=UTC&useUnicode=true&characterEncoding=utf-8
spring.datasource.username=root
spring.datasource.password=123456
# MyBatis 配置
mybatis.mapper-locations=classpath:mapper/*.xml
mybatis.type-aliases-package=com.example.demo.model
```
web.xml:
```xml
<web-app xmlns="http://java.sun.com/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee
http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd"
version="3.0">
<display-name>My Login App</display-name>
<!-- 配置 Spring MVC -->
<servlet>
<servlet-name>dispatcher</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:spring/spring-mvc.xml</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>dispatcher</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
<!-- 编码过滤器 -->
<filter>
<filter-name>encodingFilter</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<init-param>
<param-name>encoding</param-name>
<param-value>UTF-8</param-value>
</init-param>
<init-param>
<param-name>forceEncoding</param-name>
<param-value>true</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>encodingFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
</web-app>
```
spring-mvc.xml:
```xml
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc.xsd">
<!-- 开启注解扫描 -->
<context:component-scan base-package="com.example.demo"/>
<!-- 开启 Spring MVC 注解驱动 -->
<mvc:annotation-driven/>
<!-- 配置视图解析器 -->
<bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="/WEB-INF/views/"/>
<property name="suffix" value=".jsp"/>
</bean>
<!-- 配置数据源 -->
<bean id="dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="${spring.datasource.driver-class-name}"/>
<property name="url" value="${spring.datasource.url}"/>
<property name="username" value="${spring.datasource.username}"/>
<property name="password" value="${spring.datasource.password}"/>
</bean>
<!-- 配置 MyBatis -->
<bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean">
<property name="dataSource" ref="dataSource"/>
<property name="typeAliasesPackage" value="com.example.demo.model"/>
<property name="mapperLocations" value="classpath:mapper/*.xml"/>
</bean>
<bean class="org.mybatis.spring.mapper.MapperScannerConfigurer">
<property name="basePackage" value="com.example.demo.dao"/>
</bean>
<!-- 配置事务管理器 -->
<bean id="transactionManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager">
<property name="dataSource" ref="dataSource"/>
</bean>
<tx:annotation-driven transaction-manager="transactionManager"/>
</beans>
```
3. 实体类
User.java:
```java
public class User {
private int id;
private String username;
private String password;
// getter 和 setter 略
@Override
public String toString() {
return "User{" +
"id=" + id +
", username='" + username + '\'' +
", password='" + password + '\'' +
'}';
}
}
```
4. DAO 层
UserDAO.java:
```java
public interface UserDAO {
User getUserById(int id);
User getUserByUsername(String username);
void addUser(User user);
}
```
UserDAO.xml:
```xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.example.demo.dao.UserDAO">
<resultMap id="userResultMap" type="com.example.demo.model.User">
<id column="id" property="id" jdbcType="INTEGER"/>
<result column="username" property="username" jdbcType="VARCHAR"/>
<result column="password" property="password" jdbcType="VARCHAR"/>
</resultMap>
<select id="getUserById" resultMap="userResultMap">
SELECT * FROM user WHERE id = #{id}
</select>
<select id="getUserByUsername" resultMap="userResultMap">
SELECT * FROM user WHERE username = #{username}
</select>
<insert id="addUser">
INSERT INTO user (username, password) VALUES (#{username}, #{password})
</insert>
</mapper>
```
5. Service 层
UserService.java:
```java
public interface UserService {
User getUserById(int id);
User getUserByUsername(String username);
void addUser(User user);
}
```
UserServiceImpl.java:
```java
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserDAO userDAO;
@Override
public User getUserById(int id) {
return userDAO.getUserById(id);
}
@Override
public User getUserByUsername(String username) {
return userDAO.getUserByUsername(username);
}
@Override
public void addUser(User user) {
userDAO.addUser(user);
}
}
```
6. Controller 层
UserController.java:
```java
@Controller
public class UserController {
@Autowired
private UserService userService;
@RequestMapping(value = "/")
public String index() {
return "index";
}
@RequestMapping(value = "/login", method = RequestMethod.GET)
public String login() {
return "login";
}
@RequestMapping(value = "/login", method = RequestMethod.POST)
public ModelAndView login(String username, String password) {
User user = userService.getUserByUsername(username);
if (user != null && user.getPassword().equals(password)) {
ModelAndView mav = new ModelAndView("index");
mav.addObject("user", user);
return mav;
} else {
return new ModelAndView("login", "error", "用户名或密码错误!");
}
}
@RequestMapping(value = "/register", method = RequestMethod.GET)
public String register() {
return "register";
}
@RequestMapping(value = "/register", method = RequestMethod.POST)
public ModelAndView register(User user) {
userService.addUser(user);
ModelAndView mav = new ModelAndView("index");
mav.addObject("user", user);
return mav;
}
}
```
7. 视图
index.jsp:
```jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>首页</title>
</head>
<body>
<h1>欢迎您,${user.username}!</h1>
</body>
</html>
```
login.jsp:
```jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>登录</title>
</head>
<body>
<form method="post" action="/login">
<label for="username">用户名:</label>
<input type="text" id="username" name="username"><br>
<label for="password">密码:</label>
<input type="password" id="password" name="password"><br>
<input type="submit" value="登录">
</form>
<p style="color: red">${error}</p>
</body>
</html>
```
register.jsp:
```jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>注册</title>
</head>
<body>
<form method="post" action="/register">
<label for="username">用户名:</label>
<input type="text" id="username" name="username"><br>
<label for="password">密码:</label>
<input type="password" id="password" name="password"><br>
<input type="submit" value="注册">
</form>
</body>
</html>
```
以上就是一个简单的基于 Spring MVC、MySQL 和 Maven 的登录注册系统的代码示例及其完整项目结构,希望能对你有所帮助。
相关推荐
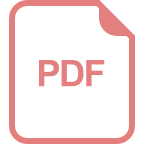
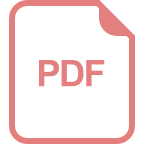
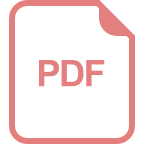












