示范一下c语言单向链表
时间: 2023-04-01 20:05:00 浏览: 123
好的,我可以回答这个问题。单向链表是一种常见的数据结构,它由一系列节点组成,每个节点包含一个数据元素和一个指向下一个节点的指针。以下是一个简单的 C 语言单向链表的示例代码:
```
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
struct Node {
int data;
struct Node* next;
};
// 创建新节点
struct Node* createNode(int data) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = data;
newNode->next = NULL;
return newNode;
}
// 在链表末尾添加新节点
void appendNode(struct Node** headRef, int data) {
struct Node* newNode = createNode(data);
if (*headRef == NULL) {
*headRef = newNode;
return;
}
struct Node* lastNode = *headRef;
while (lastNode->next != NULL) {
lastNode = lastNode->next;
}
lastNode->next = newNode;
}
// 在链表中查找指定值的节点
struct Node* findNode(struct Node* head, int data) {
struct Node* currentNode = head;
while (currentNode != NULL) {
if (currentNode->data == data) {
return currentNode;
}
currentNode = currentNode->next;
}
return NULL;
}
// 在链表中删除指定值的节点
void deleteNode(struct Node** headRef, int data) {
struct Node* currentNode = *headRef;
struct Node* prevNode = NULL;
while (currentNode != NULL) {
if (currentNode->data == data) {
if (prevNode == NULL) {
*headRef = currentNode->next;
} else {
prevNode->next = currentNode->next;
}
free(currentNode);
return;
}
prevNode = currentNode;
currentNode = currentNode->next;
}
}
// 打印链表中所有节点的值
void printList(struct Node* head) {
struct Node* currentNode = head;
while (currentNode != NULL) {
printf("%d ", currentNode->data);
currentNode = currentNode->next;
}
printf("\n");
}
// 主函数
int main() {
struct Node* head = NULL;
appendNode(&head, 1);
appendNode(&head, 2);
appendNode(&head, 3);
printList(head);
deleteNode(&head, 2);
printList(head);
struct Node* node = findNode(head, 3);
if (node != NULL) {
printf("Found node with value %d\n", node->data);
} else {
printf("Node not found\n");
}
return ;
}
```
以上是一个简单的单向链表的示例代码,包含了创建节点、添加节点、查找节点、删除节点和打印链表的功能。
阅读全文
相关推荐
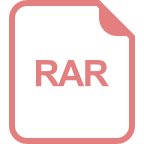
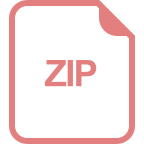
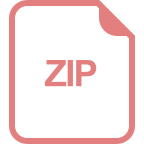
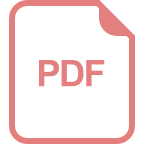
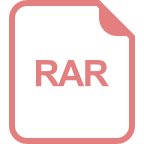
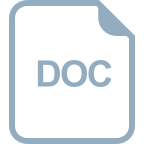
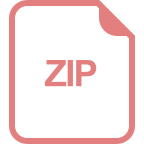
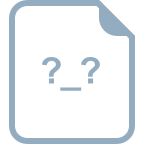
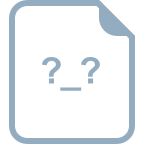
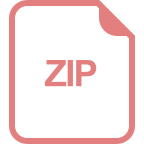
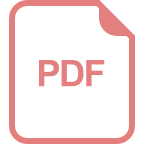
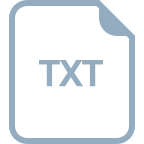
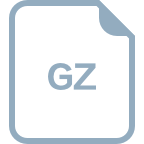