csv文件用Python读取
时间: 2023-04-05 13:04:16 浏览: 88
可以使用Python内置的csv模块来读取csv文件,具体代码如下:
```python
import csv
with open('file.csv', newline='') as csvfile:
reader = csv.reader(csvfile, delimiter=',', quotechar='"')
for row in reader:
print(', '.join(row))
```
其中,`file.csv`是要读取的csv文件名,`delimiter`参数指定分隔符,`quotechar`参数指定引号字符。读取后可以按照需要进行处理。
相关问题
csv文件用python打开是乱码
使用Python中的Pandas库可以很方便地打开CSV文件。
具体步骤如下:
1. 导入Pandas库
```python
import pandas as pd
```
2. 使用Pandas的read_csv函数读取CSV文件
```python
df = pd.read_csv('filename.csv')
```
其中,'filename.csv'为CSV文件的路径和文件名。
3. 对CSV文件进行操作
读取CSV文件后,可以对其进行各种操作,例如筛选数据、计算统计量、绘制图表等。
```python
# 筛选数据
df_filtered = df[df['column_name'] > 10]
# 计算统计量
mean_value = df['column_name'].mean()
# 绘制图表
df.plot(kind='line', x='column_name1', y='column_name2')
```
以上就是使用Python中的Pandas库打开CSV文件的基本步骤。
使用python 读取csv文件
以下是使用Python读取CSV文件的示例代码:
```python
import csv
with open('example.csv', 'r', encoding='utf-8') as csv_file:
csv_reader = csv.reader(csv_file)
for row in csv_reader:
print(row)
```
这段代码使用了Python内置的csv模块,首先使用`open()`函数打开CSV文件,然后使用`csv.reader()`函数创建一个CSV读取器对象,最后使用`for`循环逐行读取CSV文件中的数据并打印出来。
需要注意的是,`open()`函数中的第二个参数`'r'`表示以只读模式打开文件,第三个参数`encoding='utf-8'`表示使用UTF-8编码读取文件。如果CSV文件使用其他编码,需要相应地修改该参数。
相关推荐
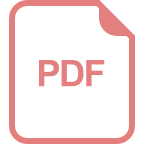












