1.请编写一个程序模拟多个售票窗口售卖一列火车票的过程。具体的,共有10个售票窗口,每个售票窗口随机休眠一段时间后售出随机数量的车票(1~10张);每张车票有唯一的编号(例如:1~1000号);请使用同步机制确保同一编号的一张票只能被售卖一次;该列车票售卖完毕,程序运行结束。 提示:可设定程序中按顺序售卖车票;请使用同步机制确保程序运行正确。
时间: 2023-06-17 13:04:33 浏览: 60
以下是使用Python实现的程序代码:
```python
import threading
import random
# 火车票总数
ticket_total = 1000
# 票号列表
ticket_list = list(range(1, ticket_total + 1))
# 锁
lock = threading.Lock()
# 售票窗口
class TicketWindow(threading.Thread):
def run(self):
while True:
# 随机休眠一段时间
time = random.randint(1, 5)
threading.Thread.sleep(time)
# 随机售出车票数量
count = random.randint(1, 10)
# 售票
for i in range(count):
lock.acquire()
if len(ticket_list) > 0:
ticket = ticket_list.pop(0)
print(f"{self.name} 售出车票 {ticket}")
lock.release()
if len(ticket_list) == 0:
return
if __name__ == "__main__":
# 创建售票窗口线程
threads = []
for i in range(10):
thread = TicketWindow()
thread.name = f"售票窗口{i + 1}"
threads.append(thread)
# 启动线程
for thread in threads:
thread.start()
# 等待线程结束
for thread in threads:
thread.join()
print("车票已售罄,程序结束。")
```
程序中使用了一个共享的票号列表,每个售票窗口线程从列表中取出一张车票进行售卖。为了防止同一票号被重复售卖,使用了锁进行同步操作。售票窗口线程随机休眠一段时间后,再随机售出一定数量的车票,直到车票售罄为止。最后程序输出“车票已售罄,程序结束。”。
相关推荐
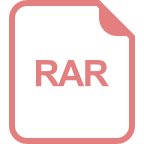
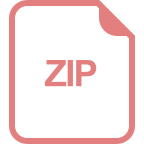






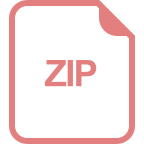
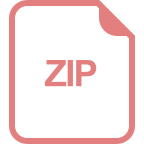
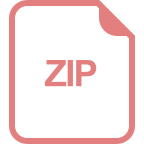
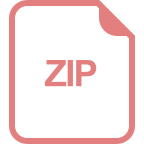
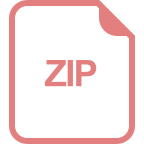
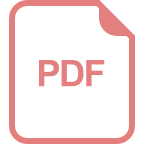
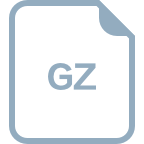
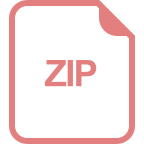