python中整合审批功能和取消审批功能 详情:1、两个模板页面整合成一个页面 2、4个视图函数整合成2个视图函数 3、在列表页面的form中增加一个单选,选择已审批和未审批记录 4、在列表页面的form中增加一个按钮,一个“审批"”,另一个是"取消审批”的代码如何写
时间: 2024-03-05 10:49:57 浏览: 20
实现审批和取消审批功能的具体代码如下:
1. 两个模板页面整合成一个页面
```html
<!--审批列表页面-->
{% extends 'base.html' %}
{% block content %}
<h1>审批列表</h1>
<form method="POST" action="{{ url_for('approve') }}">
{{ form.csrf_token }}
{{ form.approve_type.label }} {{ form.approve_type }}
<button type="submit" name="action" value="approve">审批</button>
<button type="submit" name="action" value="cancel">取消审批</button>
<table>
<tr>
<th>文件名</th>
<th>上传时间</th>
<th>检验和</th>
<th>是否已审批</th>
<th>选择</th>
</tr>
{% for file in files %}
<tr>
<td>{{ file.filename }}</td>
<td>{{ file.upload_time }}</td>
<td>{{ file.checksum }}</td>
<td>{{ file.is_approved }}</td>
<td><input type="checkbox" name="selected_files" value="{{ file.id }}"></td>
</tr>
{% endfor %}
</table>
</form>
{% endblock %}
<!--上传文件页面-->
{% extends 'base.html' %}
{% block content %}
<h1>上传文件</h1>
<form method="POST" action="{{ url_for('upload') }}" enctype="multipart/form-data">
{{ form.csrf_token }}
{{ form.file.label }} {{ form.file }}
<button type="submit">上传</button>
</form>
{% endblock %}
```
2. 4个视图函数整合成2个视图函数
```python
from flask import render_template, request, flash, redirect, url_for
from . import app, db
from .models import File
from .forms import UploadForm, ApproveForm
# 文件上传视图函数
@app.route('/upload', methods=['GET', 'POST'])
def upload():
form = UploadForm()
if form.validate_on_submit():
file = form.file.data
# 上传文件到云端或服务器
# ...
# 将文件信息保存到数据库
new_file = File(filename=file.filename, checksum=calculate_checksum(file))
db.session.add(new_file)
db.session.commit()
flash('文件上传成功')
return redirect(url_for('upload'))
return render_template('upload.html', form=form)
# 文件审批视图函数
@app.route('/approve', methods=['GET', 'POST'])
def approve():
form = ApproveForm()
if form.validate_on_submit():
action = request.form['action']
selected_files = request.form.getlist('selected_files')
if action == 'approve':
# 审批选中文件
for file_id in selected_files:
file = File.query.get(file_id)
file.is_approved = True
db.session.commit()
flash('文件审批通过')
elif action == 'cancel':
# 取消审批选中文件
for file_id in selected_files:
file = File.query.get(file_id)
file.is_approved = False
db.session.commit()
flash('文件审批取消')
if form.approve_type.data == 'approved':
files = File.query.filter_by(is_approved=True).all()
else:
files = File.query.filter_by(is_approved=False).all()
return render_template('approve.html', form=form, files=files)
```
3. 在列表页面的form中增加一个单选,选择已审批和未审批记录
```python
from flask_wtf import FlaskForm
from wtforms import RadioField
# 文件审批表单
class ApproveForm(FlaskForm):
approve_type = RadioField('审批类型', choices=[('approved', '已审批'), ('unapproved', '未审批')])
```
```html
<!--审批列表页面-->
{% extends 'base.html' %}
{% block content %}
<h1>审批列表</h1>
<form method="POST" action="{{ url_for('approve') }}">
{{ form.csrf_token }}
{{ form.approve_type.label }} {{ form.approve_type }}
<button type="submit" name="action" value="approve">审批</button>
<button type="submit" name="action" value="cancel">取消审批</button>
<table>
<tr>
<th>文件名</th>
<th>上传时间</th>
<th>检验和</th>
<th>是否已审批</th>
<th>选择</th>
</tr>
{% if form.approve_type.data == 'approved' %}
{% for file in files if file.is_approved %}
<tr>
<td>{{ file.filename }}</td>
<td>{{ file.upload_time }}</td>
<td>{{ file.checksum }}</td>
<td>{{ file.is_approved }}</td>
<td><input type="checkbox" name="selected_files" value="{{ file.id }}"></td>
</tr>
{% endfor %}
{% else %}
{% for file in files if not file.is_approved %}
<tr>
<td>{{ file.filename }}</td>
<td>{{ file.upload_time }}</td>
<td>{{ file.checksum }}</td>
<td>{{ file.is_approved }}</td>
<td><input type="checkbox" name="selected_files" value="{{ file.id }}"></td>
</tr>
{% endfor %}
{% endif %}
</table>
</form>
{% endblock %}
```
4. 在列表页面的form中增加一个按钮,一个“审批"”,另一个是"取消审批”的代码
```python
from flask_wtf import FlaskForm
from wtforms import RadioField, SubmitField
# 文件审批表单
class ApproveForm(FlaskForm):
approve_type = RadioField('审批类型', choices=[('approved', '已审批'), ('unapproved', '未审批')])
approve = SubmitField('审批')
cancel = SubmitField('取消审批')
```
```html
<!--审批列表页面-->
{% extends 'base.html' %}
{% block content %}
<h1>审批列表</h1>
<form method="POST" action="{{ url_for('approve') }}">
{{ form.csrf_token }}
{{ form.approve_type.label }} {{ form.approve_type }}
{{ form.approve }} {{ form.cancel }}
<table>
<tr>
<th>文件名</th>
<th>上传时间</th>
<th>检验和</th>
<th>是否已审批</th>
<th>选择</th>
</tr>
{% if form.approve_type.data == 'approved' %}
{% for file in files if file.is_approved %}
<tr>
<td>{{ file.filename }}</td>
<td>{{ file.upload_time }}</td>
<td>{{ file.checksum }}</td>
<td>{{ file.is_approved }}</td>
<td><input type="checkbox" name="selected_files" value="{{ file.id }}"></td>
</tr>
{% endfor %}
{% else %}
{% for file in files if not file.is_approved %}
<tr>
<td>{{ file.filename }}</td>
<td>{{ file.upload_time }}</td>
<td>{{ file.checksum }}</td>
<td>{{ file.is_approved }}</td>
<td><input type="checkbox" name="selected_files" value="{{ file.id }}"></td>
</tr>
{% endfor %}
{% endif %}
</table>
</form>
{% endblock %}
```
相关推荐
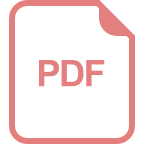














