1)定义一个类 Person; 2)在类中定义属性:姓名、性别、年龄、学历、身高。 3)对属性实现封装,并编写各属性的读写访问器。对属性中的年龄进行控制,要求年龄大于0,否则使用默认值代替(年龄默认 18)。 4)在类中定义show (),功能为输出属性信息。 5)在main方法中实例化2个对象,通过读写访问器完成对属性的赋值和输出操作。
时间: 2023-05-28 12:04:25 浏览: 247
1) class Person {
2) private String name;
3) private String gender;
4) private int age;
5) private String education;
6) private double height;
7)
8) public String getName() {
9) return name;
10) }
11) public void setName(String name) {
12) this.name = name;
13) }
14) public String getGender() {
15) return gender;
16) }
17) public void setGender(String gender) {
18) this.gender = gender;
19) }
20) public int getAge() {
21) return age;
22) }
23) public void setAge(int age) {
24) if(age > 0) {
25) this.age = age;
26) } else {
27) this.age = 18;
28) }
29) }
30) public String getEducation() {
31) return education;
32) }
33) public void setEducation(String education) {
34) this.education = education;
35) }
36) public double getHeight() {
37) return height;
38) }
39) public void setHeight(double height) {
40) this.height = height;
41) }
42)
43) public void show() {
44) System.out.println("姓名:" + this.name);
45) System.out.println("性别:" + this.gender);
46) System.out.println("年龄:" + this.age);
47) System.out.println("学历:" + this.education);
48) System.out.println("身高:" + this.height);
49) }
50) }
51)
52) public class Main {
53) public static void main(String[] args) {
54) Person p1 = new Person();
55) p1.setName("张三");
56) p1.setGender("男");
57) p1.setAge(25);
58) p1.setEducation("本科");
59) p1.setHeight(180);
60) p1.show();
61)
62) Person p2 = new Person();
63) p2.setName("李四");
64) p2.setGender("女");
65) p2.setAge(-5);
66) p2.setEducation("硕士");
67) p2.setHeight(170);
68) p2.show();
69) }
70) }
阅读全文
相关推荐
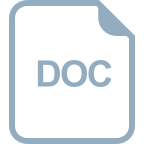
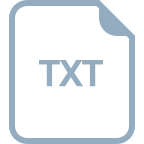
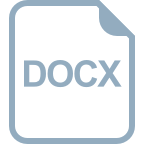















