解析一下const obj = { 2: 5, 3: 6, 4: 7 } obj[Symbol.iterator] = function () { return { next: function () { if (this._countDown === 3) { return { value: this._countDown, done: true } } this._countDown = this._countDown + 1 return { value: obj[this._countDown], done: false } }, _countDown: 0, } } for (const i of obj) { console.log(i) }
时间: 2024-04-23 15:23:01 浏览: 26
这段代码中定义了一个名为 `obj` 的对象,它有三个属性 `2`、`3` 和 `4`,分别对应着值 `5`、`6` 和 `7`。然后通过 `obj[Symbol.iterator]` 方法给 `obj` 对象添加了一个迭代器,这个迭代器包含一个 `_countDown` 属性和一个 `next` 方法。迭代器的 `next` 方法会根据 `_countDown` 属性的值返回下一个迭代的对象,其中 `value` 属性为 `obj` 对象中下一个键对应的值,`done` 属性表示是否已经迭代完成。当 `_countDown` 属性的值为 `3` 时,迭代器返回 `{ value: this._countDown, done: true }`,表示迭代已经完成。否则,迭代器会将 `_countDown` 属性的值加 1,然后返回 `{ value: obj[this._countDown], done: false }`,表示迭代还未完成。最后,使用 `for...of` 循环遍历 `obj` 对象,循环内部通过迭代器的 `next` 方法获取下一个值并输出。输出的结果为 `5`、`6` 和 `7`。
相关问题
nvalid attempt to destructure non-iterable instance. In order to be iterable, non-array objects must have a [Symbol.iterator]() method.
这个错误通常是在尝试解构非可迭代实例时出现的。解构是将数组或对象中的值分配给变量的过程。如果你尝试对非数组或非可迭代对象进行解构,就会出现这个错误。为了解决这个问题,你需要确保你的对象是可迭代的,或者使用其他方法来访问对象的属性。
以下是一些解决方法:
1.确保你的对象是可迭代的,例如数组或具有[Symbol.iterator]方法的对象。
2.使用对象的属性来访问它的值,而不是尝试解构它。
3.使用try-catch语句来捕获错误并处理它们。
```javascript
// 示例代码
const obj = { name: 'John', age: 30 };
const { name, age } = obj; // 正确的解构方式
const obj2 = { name: 'John', age: 30 };
for (let key in obj2) {
console.log(obj2[key]); // 使用对象的属性来访问它的值
}
const obj3 = { name: 'John', age: 30 };
try {
const { name, age } = obj3;
} catch (e) {
console.log('Error:', e.message); // 使用try-catch语句来捕获错误并处理它们
}
```
macchina.io如何通过C++解析JSON格式数据知道其有哪些键并将它们复制给一个vector<std::string>对象
在macchina.io中,可以使用Poco C++库中的JSON解析器来解析JSON格式数据,并使用Poco::Dynamic::Var类来获取JSON对象中的所有键名,并将它们复制给一个vector<std::string>对象。
下面是一个示例代码:
```c++
#include <vector>
#include <string>
#include "Poco/JSON/Parser.h"
#include "Poco/Dynamic/Var.h"
using namespace Poco::JSON;
// JSON格式数据
std::string jsonStr = "{\"name\":\"John\",\"age\":30,\"city\":\"New York\"}";
// 解析JSON数据
Parser parser;
Var result = parser.parse(jsonStr);
Object::Ptr obj = result.extract<Object::Ptr>();
// 获取所有键名
const Object::NameVec& keys = obj->getNames();
std::vector<std::string> keyList;
for (Object::NameVec::const_iterator it = keys.begin(); it != keys.end(); ++it)
{
keyList.push_back(*it);
}
```
在上面的示例中,我们使用Poco::JSON::Parser类来解析JSON格式的字符串,然后将结果存储在Poco::Dynamic::Var类型的变量中。使用Poco::Dynamic::Var::extract<Object::Ptr>()方法将结果转换为Poco::JSON::Object::Ptr类型的指针,然后使用Poco::JSON::Object::getNames()方法获取JSON对象中的所有键名,并将它们复制到一个vector<std::string>对象中。
相关推荐
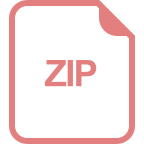
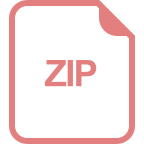
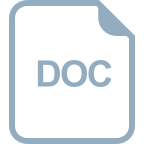












