计算机网络中GBN协议算法具体的C-Sharp代码
时间: 2024-04-29 07:22:56 浏览: 75
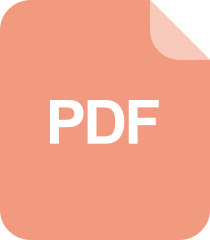
计算机网络原理GBN协议代码.pdf

以下是GBN协议的C#代码实现:
```
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Net;
using System.Net.Sockets;
namespace GBNProtocol
{
class GBNClient
{
private const int TIMEOUT = 5000;
private const int WINDOW_SIZE = 4;
private const int BUFFER_SIZE = 256;
private const int SERVER_PORT = 8888;
private const string SERVER_IP = "127.0.0.1";
private static int lastAck = 0;
private static int lastSent = 0;
private static int lastReceived = -1;
static void Main(string[] args)
{
byte[][] message = new byte[WINDOW_SIZE][];
bool[] acks = new bool[WINDOW_SIZE];
IPEndPoint serverEndpoint = new IPEndPoint(IPAddress.Parse(SERVER_IP), SERVER_PORT);
Socket clientSocket = new Socket(AddressFamily.InterNetwork, SocketType.Dgram, ProtocolType.Udp);
Console.Write("Enter message to send: ");
string input = Console.ReadLine();
byte[] data = Encoding.ASCII.GetBytes(input);
while (lastAck < data.Length)
{
for (int i = lastSent; i <= Math.Min(lastSent + WINDOW_SIZE - 1, data.Length - 1); i++)
{
message[i % WINDOW_SIZE] = new byte[BUFFER_SIZE];
data.CopyTo(message[i % WINDOW_SIZE], 0);
clientSocket.SendTo(message[i % WINDOW_SIZE], serverEndpoint);
lastSent++;
}
clientSocket.ReceiveTimeout = TIMEOUT;
byte[] buffer = new byte[BUFFER_SIZE];
try
{
EndPoint remoteEndPoint = new IPEndPoint(IPAddress.Any, 0);
int bytesReceived = clientSocket.ReceiveFrom(buffer, ref remoteEndPoint);
if (bytesReceived > 0)
{
string ackString = Encoding.ASCII.GetString(buffer, 0, bytesReceived);
int ack = Int32.Parse(ackString);
if (ack > lastAck && ack <= lastSent)
{
for (int i = lastAck + 1; i <= ack; i++)
{
acks[(i - 1) % WINDOW_SIZE] = true;
}
lastAck = ack;
}
}
}
catch (SocketException e)
{
Console.WriteLine("Timeout. Resending packets...");
continue;
}
if (acks[lastReceived % WINDOW_SIZE])
{
lastReceived++;
}
Array.Clear(message[lastReceived % WINDOW_SIZE], 0, BUFFER_SIZE);
acks[lastReceived % WINDOW_SIZE] = false;
}
clientSocket.Close();
Console.WriteLine("All packets sent and acknowledged.");
Console.ReadKey();
}
}
}
```
阅读全文
相关推荐
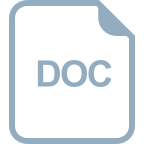

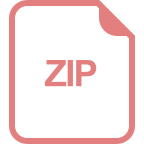
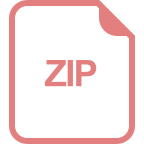
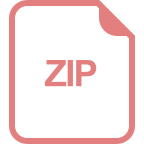
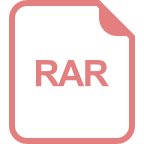
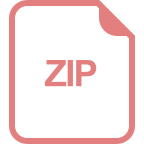
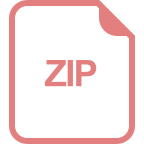
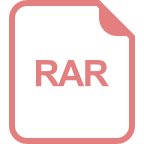
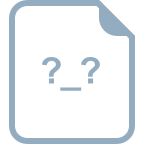
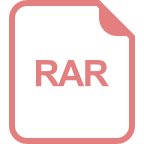
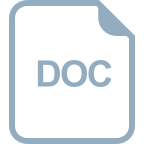
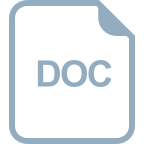
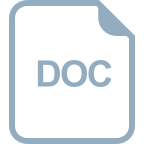

