php base64上传
时间: 2024-10-17 22:01:32 浏览: 18
在PHP中,Base64编码常用于文件上传处理,特别是在需要将大文件转换成字符串形式,以便于通过URL传递或者存储时。当你上传文件时,可以先读取文件内容,然后使用`base64_encode()`函数将其转换为Base64格式。以下是基本步骤:
1. **打开文件流**:
```php
$file = fopen("path/to/your/file", "rb");
```
2. **读取文件内容**:
```php
$file_content = fread($file, filesize("path/to/your/file"));
```
3. **Base64编码**:
```php
$encoded_content = base64_encode($file_content);
```
4. **保存Base64字符串**:
可能会在数据库中存储这个字符串,或者作为表单字段值发送到服务器。
5. **接收并解码**:
当需要从服务器获取这个Base64数据时,接收后使用`base64_decode()`函数进行解码:
```php
$decoded_content = base64_decode($_POST['file_base64']);
file_put_contents("path/to/save/received/file", $decoded_content);
```
相关问题
帮我写一点php base64 文件上传代码
好的,请参考以下代码:
```php
<?php
if(isset($_POST['submit'])){
$file = $_FILES['file'];
$filename = $file['name'];
$fileTmpName = $file['tmp_name'];
$fileSize = $file['size'];
$fileError = $file['error'];
$fileType = $file['type'];
$fileExt = explode('.', $filename);
$fileActualExt = strtolower(end($fileExt));
$allowed = array('jpg', 'jpeg', 'png', 'pdf');
if(in_array($fileActualExt, $allowed)){
if($fileError === 0){
if($fileSize < 1000000){
$fileContent = file_get_contents($fileTmpName);
$base64 = base64_encode($fileContent);
// 上传到服务器或储存到数据库中
echo "文件上传成功!";
} else {
echo "文件过大!";
}
} else {
echo "上传文件出错!";
}
} else {
echo "不支持该文件类型!";
}
}
?>
<form action="" method="post" enctype="multipart/form-data">
<input type="file" name="file">
<button type="submit" name="submit">上传</button>
</form>
```
这段代码实现了文件上传,并将文件内容转为 base64 编码。你可以将它上传到服务器或储存到数据库中。注意,这只是一个基础的示例代码,你需要根据你的实际需求进行修改。
用PHP写一个base64编码上传的网络访问
以下是一个使用 PHP 实现的 base64 编码上传的网络访问代码示例:
```php
<?php
$base64_image = 'data:image/jpeg;base64,/9j/4AAQSkZJRg...'; // base64 编码的图片数据
$url = 'https://example.com/upload.php'; // 上传接口的 URL
// 将 base64 编码的图片数据解码为二进制数据
$image_data = base64_decode(preg_replace('#^data:image/\w+;base64,#i', '', $base64_image));
// 创建 CURL 请求并设置参数
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $image_data);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, false);
// 发送 CURL 请求并获取响应
$response = curl_exec($ch);
// 输出响应结果
echo $response;
// 关闭 CURL 请求
curl_close($ch);
?>
```
在上面的示例中,我们首先将 base64 编码的图片数据解码为二进制数据,然后使用 CURL 发送 POST 请求并将二进制数据作为请求体发送到指定的上传接口。最后,我们获取响应结果并输出。需要注意的是,由于这是一个上传操作,我们需要将 `CURLOPT_POST` 参数设置为 `true`,并将二进制数据作为 `CURLOPT_POSTFIELDS` 参数的值。
阅读全文
相关推荐
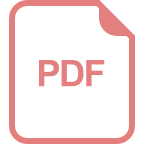
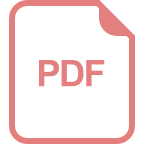
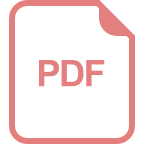
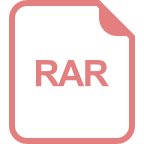
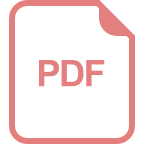
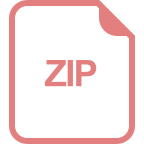
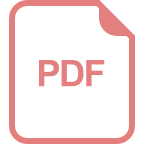
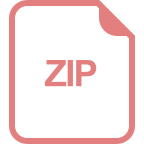
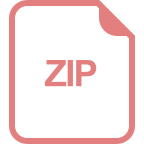
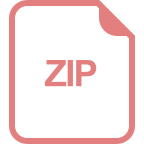
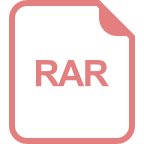
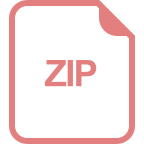


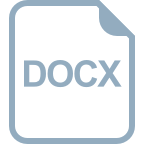