pytorch图像验证码识别模型并测试代码
时间: 2023-07-29 13:10:54 浏览: 156
以下是一个基于PyTorch的图像验证码识别模型及测试代码的示例:
```python
import torch
import torchvision.transforms as transforms
from PIL import Image
# 定义模型结构
class CaptchaModel(torch.nn.Module):
def __init__(self):
super(CaptchaModel, self).__init__()
self.conv1 = torch.nn.Conv2d(3, 32, kernel_size=3, stride=1, padding=1)
self.pool1 = torch.nn.MaxPool2d(kernel_size=2, stride=2, padding=0)
self.conv2 = torch.nn.Conv2d(32, 64, kernel_size=3, stride=1, padding=1)
self.pool2 = torch.nn.MaxPool2d(kernel_size=2, stride=2, padding=0)
self.conv3 = torch.nn.Conv2d(64, 128, kernel_size=3, stride=1, padding=1)
self.pool3 = torch.nn.MaxPool2d(kernel_size=2, stride=2, padding=0)
self.fc1 = torch.nn.Linear(128*4*13, 1024)
self.fc2 = torch.nn.Linear(1024, 4*10)
def forward(self, x):
x = self.conv1(x)
x = torch.nn.functional.relu(x)
x = self.pool1(x)
x = self.conv2(x)
x = torch.nn.functional.relu(x)
x = self.pool2(x)
x = self.conv3(x)
x = torch.nn.functional.relu(x)
x = self.pool3(x)
x = x.view(-1, 128*4*13)
x = self.fc1(x)
x = torch.nn.functional.relu(x)
x = self.fc2(x)
return x
# 加载模型
model = CaptchaModel()
model.load_state_dict(torch.load('captcha_model.pth'))
# 定义验证码字符集
charset = '0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ'
# 定义预处理函数
preprocess = transforms.Compose([
transforms.Resize((50, 200)),
transforms.ToTensor(),
])
# 定义测试函数
def test_captcha(image_path):
# 加载图像
image = Image.open(image_path).convert('RGB')
# 预处理图像
image = preprocess(image)
# 扩展维度
image = image.unsqueeze(0)
# 预测结果
output = model(image)
# 获取预测结果的索引
output = torch.argmax(output, dim=1)
# 将索引转换成字符
captcha = ''.join([charset[i] for i in output])
return captcha
# 测试
captcha = test_captcha('captcha.png')
print(captcha)
```
需要注意的是,在运行代码之前,需要先准备好验证码图像,并将其保存为`captcha.png`文件。如果需要识别多张验证码图像,只需要在测试函数中添加一个循环即可。
此外,需要提醒的是,这只是一个简单的验证码识别模型,针对不同的验证码类型,可能需要进行一些调整,比如修改模型结构、调整字符集等。
阅读全文
相关推荐
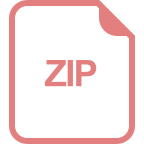

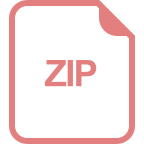



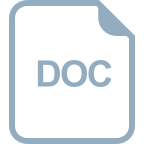
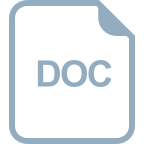
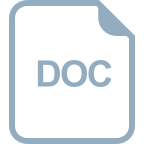
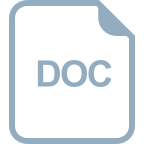
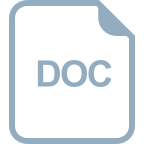
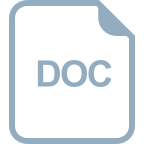



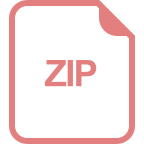
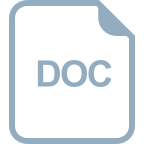