并联机器人门型轨迹编程
时间: 2023-09-17 11:10:16 浏览: 115
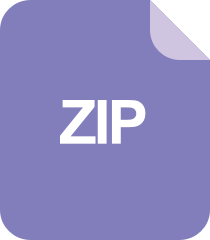
并联机器人控制程序

并联机器人门型轨迹编程需要考虑以下几个步骤:
1. 确定门型轨迹的运动规律和路径,通常可以使用轨迹规划算法来实现,比如三次样条插值、贝塞尔曲线等。
2. 确定机器人各关节的角度变化规律,可以使用逆向运动学算法来实现。
3. 将机器人关节角度与门型轨迹的运动规律相结合,生成机器人的运动轨迹。
4. 将生成的运动轨迹转化为机器人控制指令,以实现机器人的自动化控制。
下面是一个简单的示例代码,用于实现一个四自由度的并联机器人的门型轨迹编程:
```python
#-*- coding:utf-8 -*-
import numpy as np
import matplotlib.pyplot as plt
# 机器人参数
L1 = 300.0
L2 = 150.0
L3 = 100.0
L4 = 80.0
# 门型轨迹参数
delta = 100.0 # 门型轨迹高度
T = 10.0 # 门型轨迹周期
# 逆向运动学求解函数
def inv_kinematics(x, y, z):
theta1 = np.arctan2(y, x)
d = np.sqrt(x*x + y*y)
alpha = np.arctan2(z - L1, d)
beta = np.arccos((L2*L2 + d*d + (z-L1)*(z-L1) - L3*L3) / (2*L2*np.sqrt(d*d + (z-L1)*(z-L1))))
theta2 = alpha - beta
gamma = np.arccos((L2*L2 + L3*L3 - d*d - (z-L1)*(z-L1)) / (2*L2*L3))
theta3 = np.pi - gamma
theta4 = np.arctan2(0, -1)
return [theta1, theta2, theta3, theta4]
# 生成门型轨迹
def generate_trajectory():
t = np.linspace(0, T, 1000)
x = 0.5 * delta * np.sin(2*np.pi/T*t)
y = 0.5 * delta * np.sin(4*np.pi/T*t)
z = L1 + 0.5 * delta * np.cos(2*np.pi/T*t)
return x, y, z
# 运动规划
def motion_planning():
x, y, z = generate_trajectory()
thetas = []
for i in range(len(x)):
theta = inv_kinematics(x[i], y[i], z[i])
thetas.append(theta)
return np.array(thetas)
# 绘制机器人运动轨迹
def plot_trajectory(thetas):
T = len(thetas)
x = np.zeros(T)
y = np.zeros(T)
z = np.zeros(T)
for i in range(T):
theta = thetas[i]
x[i] = L2*np.sin(theta[1]) + L3*np.sin(theta[1]+theta[2]) + L4*np.sin(theta[1]+theta[2]+theta[3])
y[i] = L2*np.cos(theta[1]) + L3*np.cos(theta[1]+theta[2]) + L4*np.cos(theta[1]+theta[2]+theta[3])
z[i] = L1 + L2*np.sin(theta[0]) + L3*np.sin(theta[0]) + L4*np.sin(theta[0])
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.plot(x, y, z, 'b-')
ax.set_xlabel('x')
ax.set_ylabel('y')
ax.set_zlabel('z')
ax.set_xlim([-500, 500])
ax.set_ylim([-500, 500])
ax.set_zlim([0, 500])
plt.show()
# 主函数
if __name__ == '__main__':
thetas = motion_planning()
plot_trajectory(thetas)
```
运行以上代码后,会生成一个机器人的门型轨迹运动动画。其中,`inv_kinematics()` 函数用于求解逆向运动学,`generate_trajectory()` 函数用于生成门型轨迹,`motion_planning()` 函数用于运动规划,`plot_trajectory()` 函数用于绘制机器人的运动轨迹。
阅读全文
相关推荐
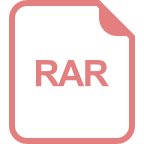

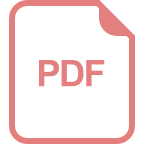
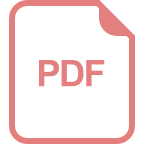
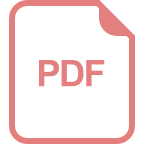
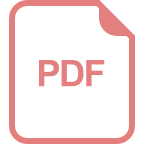
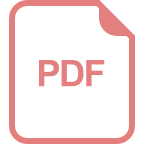
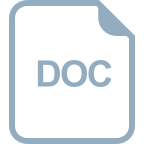
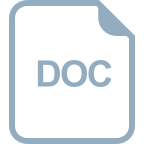







