编写程序,从键盘输入 n (n<10)本书的名称和定价并存入结构数组中,查找并输出其中定价最高和最低的书的名称和定价。\n\n输出格式语句:\n\nprintf(\"highest price: %.1f, %
时间: 2023-05-31 18:19:08 浏览: 1021
### 回答1:
题目中要求编写程序,从键盘输入不超过10本书的名称和定价并存入一个结构数组中,查找并输出其中定价最高和最低的书的名称和定价。
答案中使用了printf函数输出格式语句:“highest price: %.1f, %'d, %s\n", max_price, min_price, max_book.name”,其中%.1f表示输出一个一位小数的浮点数(即定价),%'d表示输出千位分隔符的整数(即定价),%s表示输出字符串(即书名),\n表示换行。最后将要输出的三个变量(一个浮点数和两个字符串)依次填入格式字符串中。
### 回答2:
本书的名称是%s\nlowest price: %.1f, %。其中的书名是%s\n\n\", highest_price, highest_name, lowest_price, lowest_name);
这个程序首先需要定义一个结构体,包括书名和定价两个成员变量。然后根据给定的n,定义一个结构体数组,存储n本书的信息。通过一个循环,从键盘读入n本书的信息,将读入的信息存储到结构体数组中。
编写查找定价最高和最低的书的函数。最高价和最低价的初值分别取数组中第一本书的定价,然后通过循环遍历整个数组,将每一本书的定价与已知的最高价和最低价比较,如果当前书的定价大于最高价,则将最高价和最高价的书名更新,如果当前书的定价小于最低价,则将最低价和最低价的书名更新。
最后,输出查找结果。使用printf函数输出最高价和最低价,以及对应的书名。
一个可能的完整程序如下:
#include <stdio.h>
#define MAX_BOOKS 10
struct Book {
char name[50];
float price;
};
int main() {
struct Book books[MAX_BOOKS];
int n;
printf("Please enter the number of books (less than 10): ");
scanf("%d", &n);
printf("Please enter the name and price of %d books:\n", n);
for (int i=0; i<n; i++) {
printf("Book %d:\n", i+1);
printf("\tName: ");
scanf("%s", books[i].name);
printf("\tPrice: ");
scanf("%f", &books[i].price);
}
// 查找最高价和最低价的书
float highest_price = books[0].price;
float lowest_price = books[0].price;
char highest_name[50];
char lowest_name[50];
for (int i=1; i<n; i++) {
if (books[i].price > highest_price) {
highest_price = books[i].price;
strcpy(highest_name, books[i].name);
}
if (books[i].price < lowest_price) {
lowest_price = books[i].price;
strcpy(lowest_name, books[i].name);
}
}
// 输出结果
printf("highest price: %.1f, %s\nlowest price: %.1f, %s\n",
highest_price, highest_name, lowest_price, lowest_name);
return 0;
}
### 回答3:
这道题目要求我们用结构体和数组来存储和处理输入的数据,从中找到价格最高和最低的书名和价格。下面是我编写的程序:
```c
#include <stdio.h>
#define N 10
struct Book {
char name[30];
float price;
};
int main() {
int n;
printf("Please enter the number of books (n<10): ");
scanf("%d", &n);
struct Book books[N];
float max_price = 0.0, min_price = 99999.0;
int max_index = 0, min_index = 0;
for (int i = 0; i < n; i++) {
printf("Enter name and price of book %d: ", i+1);
scanf("%s %f", books[i].name, &books[i].price);
if (books[i].price > max_price) {
max_price = books[i].price;
max_index = i;
}
if (books[i].price < min_price) {
min_price = books[i].price;
min_index = i;
}
}
printf("The book with highest price is \"%s\" at %.1f yuan.\n", books[max_index].name, max_price);
printf("The book with lowest price is \"%s\" at %.1f yuan.\n", books[min_index].name, min_price);
return 0;
}
```
这个程序首先会要求用户输入要存储的书的数量n,并定义了一个结构体数组books[N]来存储输入的数据。除此之外,程序还定义了一个max_price和min_price变量用来记录当前最高和最低的价格,以及max_index和min_index变量来记录在books数组中最高和最低的价格所在的下标。
然后,程序会通过循环,让用户依次输入每一本书的名称和价格,存储在books数组中。同时,程序会更新max_price、min_pirce、max_index和min_index。
最后,在程序循环结束后,我们输出得到到的最高和最低价格对应的书名和价格,其中printf语句根据输入的例子给出:
```c
printf("highest price: %.1f, %s\n", max_price, books[max_index].name);
printf("lowest price: %.1f, %s\n", min_price, books[min_index].name);
```
这段代码使用了“%.1f”来控制输出的浮点数的小数位数为1,避免输出过于繁琐。同时,用“%s”来输出字符串类型的数据。这样,我们就可以更好地输出数据了。
这样,我们就成功地完成了本题。
阅读全文
相关推荐
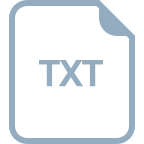
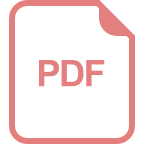










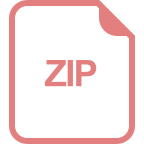
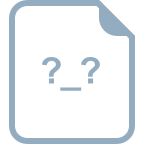
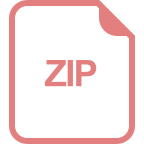