爬取网页数据并导入mysql数据库
时间: 2023-11-11 09:49:48 浏览: 84
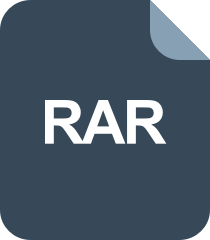
爬取数据并存储到mysql
可以使用Python中的BeautifulSoup库来解析网页数据,并使用Python中的MySQLdb库将数据导入MySQL数据库。
首先,需要安装MySQLdb库和BeautifulSoup库。可以使用以下命令进行安装:
```
pip install mysqlclient
pip install beautifulsoup4
```
接下来,使用Python中的requests库获取网页数据,并使用BeautifulSoup库解析数据:
```python
import requests
from bs4 import BeautifulSoup
url = 'https://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# 解析数据并保存到变量中,例如:
title = soup.title.string
content = soup.find('div', {'class': 'content'}).text
```
最后,使用MySQLdb库连接MySQL数据库,并将数据插入到数据库中:
```python
import MySQLdb
# 连接数据库
conn = MySQLdb.connect(
host='localhost',
user='root',
password='password',
db='database_name'
)
# 获取游标
cursor = conn.cursor()
# 插入数据
sql = "INSERT INTO table_name (title, content) VALUES (%s, %s)"
values = (title, content)
cursor.execute(sql, values)
# 提交事务并关闭连接
conn.commit()
conn.close()
```
注意:以上代码仅为示例,具体的网页结构和数据库表结构需要根据实际情况进行修改。
阅读全文
相关推荐
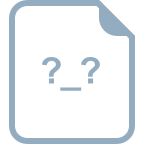
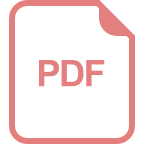

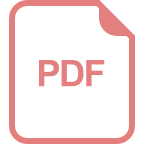
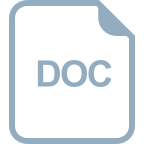
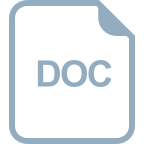
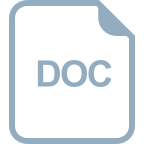
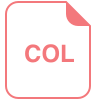
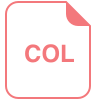
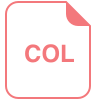




