import mnist_loader training_data, validation_data, test_data = \ mnist_loader.load_data_wrapper()
时间: 2023-09-17 22:08:30 浏览: 55
好的,我明白了。关于你的问题,这是一些Python代码,用于加载mnist数据集并准备训练、验证和测试数据。mnist_loader是一个自定义的Python模块,其中定义了load_data_wrapper函数,它将原始mnist数据集转换为适合前馈神经网络训练的格式。训练数据、验证数据和测试数据都是由包含输入和正确输出的元组组成的列表。在这段代码中,使用load_data_wrapper函数将原始mnist数据集导入,三个数据集分别赋值给training_data、validation_data和test_data。
相关问题
from tensorflow.examples.tutorials.mnist import input_data
`from tensorflow.examples.tutorials.mnist import input_data`是导入TensorFlow中的MNIST数据集的模块。这个模块可以帮助我们加载和处理MNIST数据集,以便在机器学习和深度学习任务中使用。
以下是一个使用`input_data`模块加载MNIST数据集的示例代码:
```python
from tensorflow.examples.tutorials.mnist import input_data
# 使用one-hot编码加载MNIST数据集
mnist = input_data.read_data_sets("MNIST_data/", one_hot=True)
# 打印测试集的形状
print('测试集shape:', mnist.test.images.shape)
```
这段代码首先从`tutorials.mnist`模块中导入`input_data`函数。然后,使用`read_data_sets`函数从指定的路径加载MNIST数据集,并将其存储在`mnist`对象中。最后,我们打印出测试集的形状,以便确认数据加载成功。
tf.keras.datasets.mnist.load_data
tf.keras.datasets.mnist.load_data是一个tensorflow中的函数,用于加载并返回MNIST数据集。
MNIST是一个非常经典的手写数字数据集,其中包含了60000个训练样本和10000个测试样本。该数据集被广泛用于机器学习和深度学习的训练和验证。
load_data()函数会自动下载MNIST数据集到本地,并将其分为训练集和测试集。下载完成后,load_data()函数会将训练集和测试集分别存储在两个元组中。
训练集元组包含两个NumPy数组:(x_train, y_train)。x_train包含了60000个28x28的灰度图像,每个图像代表一个手写数字样本。y_train包含了与x_train对应的60000个标签,取值范围为0到9,表示手写数字的类别。
测试集元组也包含两个NumPy数组:(x_test, y_test)。x_test包含了10000个28x28的灰度图像,每个图像代表一个手写数字样本。y_test包含了与x_test对应的10000个标签。
load_data()函数的返回结果是一个元组,其中包含了训练集元组和测试集元组。我们可以通过如下方式获取这些数据:
(x_train, y_train), (x_test, y_test) = tf.keras.datasets.mnist.load_data()
加载MNIST数据集后,我们就可以使用这些数据进行模型的训练和测试,用于识别手写数字。
相关推荐
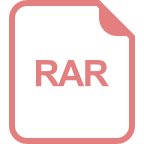
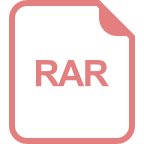












