java 生成hostkey
时间: 2023-10-06 18:05:17 浏览: 80
如果你想在Java中生成SSH的Host Key,可以使用Bouncy Castle库来实现。
以下是一个使用Bouncy Castle生成SSH Host Key的示例代码:
```java
import java.io.IOException;
import java.security.KeyPair;
import java.security.KeyPairGenerator;
import java.security.NoSuchAlgorithmException;
import org.bouncycastle.asn1.pkcs.PrivateKeyInfo;
import org.bouncycastle.asn1.x509.AlgorithmIdentifier;
import org.bouncycastle.asn1.pkcs.PKCSObjectIdentifiers;
import org.bouncycastle.crypto.params.RSAKeyParameters;
import org.bouncycastle.crypto.util.PrivateKeyFactory;
import org.bouncycastle.openssl.jcajce.JcaPEMWriter;
import org.bouncycastle.openssl.PEMWriter;
public class HostKeyGenerator {
public static void main(String[] args) throws NoSuchAlgorithmException, IOException {
// 使用RSA算法生成KeyPairGenerator对象
KeyPairGenerator keyPairGenerator = KeyPairGenerator.getInstance("RSA");
// 初始化KeyPairGenerator对象,指定密钥长度为2048位
keyPairGenerator.initialize(2048);
// 生成密钥对
KeyPair keyPair = keyPairGenerator.generateKeyPair();
// 获取私钥
RSAKeyParameters privateKeyParams = (RSAKeyParameters) PrivateKeyFactory.createKey(keyPair.getPrivate().getEncoded());
// 指定算法标识符
AlgorithmIdentifier algorithmIdentifier = new AlgorithmIdentifier(PKCSObjectIdentifiers.rsaEncryption, privateKeyParams);
// 生成PrivateKeyInfo对象
PrivateKeyInfo privateKeyInfo = new PrivateKeyInfo(algorithmIdentifier, keyPair.getPrivate().getEncoded());
// 输出私钥的PEM格式
try (PEMWriter pemWriter = new JcaPEMWriter(new java.io.OutputStreamWriter(System.out))) {
pemWriter.writeObject(privateKeyInfo);
}
}
}
```
注意,在输出私钥的PEM格式时,这里使用了Bouncy Castle库中的PEMWriter和JcaPEMWriter类。在实际使用中,你需要根据具体的需求选择合适的方式来保存和使用SSH Host Key。
相关推荐
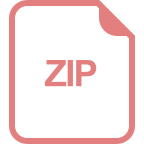
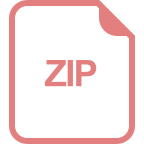
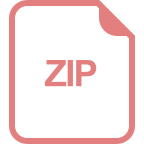














