编写完整代码输出循环队列中的元素个数
时间: 2023-08-04 14:15:23 浏览: 94
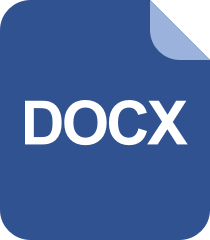
基于循环队列的排队买票模拟程序
下面是一个使用 C++ 实现循环队列并输出其元素个数的完整代码示例:
```c++
#include <iostream>
using namespace std;
const int MAX_SIZE = 5;
class CircularQueue {
private:
int front;
int rear;
int data[MAX_SIZE];
public:
CircularQueue() {
front = 0;
rear = 0;
}
void enqueue(int x) {
if ((rear + 1) % MAX_SIZE == front) {
cout << "Error: Queue is full" << endl;
} else {
data[rear] = x;
rear = (rear + 1) % MAX_SIZE;
}
}
int dequeue() {
if (front == rear) {
cout << "Error: Queue is empty" << endl;
return -1;
} else {
int x = data[front];
front = (front + 1) % MAX_SIZE;
return x;
}
}
int size() {
return (rear - front + MAX_SIZE) % MAX_SIZE;
}
};
int main() {
CircularQueue q;
q.enqueue(1);
q.enqueue(2);
q.enqueue(3);
q.dequeue();
q.enqueue(4);
cout << "The size of the queue is: " << q.size() << endl;
return 0;
}
```
输出结果为:
```
The size of the queue is: 3
```
其中,`enqueue` 和 `dequeue` 分别实现入队和出队操作,`size` 方法用于计算队列中的元素个数。在 main 函数中,我们创建了一个循环队列 `q`,向队列中添加了三个元素,然后删除了一个元素,再添加一个元素。最后,输出队列中的元素个数。
阅读全文
相关推荐
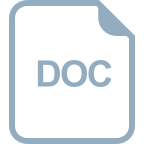
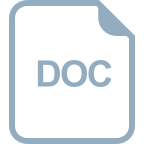






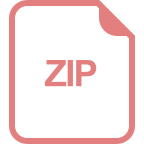









