用canvas写一个股票路线上升的动态路径特效,带箭头
时间: 2023-05-15 22:07:09 浏览: 116
可以使用 canvas 的 API 来实现股票路线上升的动态路径特效,具体步骤如下:
1. 创建一个 canvas 元素,并设置其宽度和高度。
2. 获取 canvas 的上下文对象,使用该对象来绘制图形。
3. 绘制股票路线的路径,可以使用 moveTo 和 lineTo 方法来绘制直线段,使用 quadraticCurveTo 和 bezierCurveTo 方法来绘制曲线段。
4. 绘制箭头,可以使用 moveTo 和 lineTo 方法来绘制箭头的线段,使用 fill 方法来填充箭头的三角形部分。
5. 使用 setInterval 方法来定时更新股票路线的路径,每次更新时,先清除 canvas 上的所有内容,然后重新绘制路径和箭头。
以下是示例代码:
```html
<canvas id="canvas" width="400" height="300"></canvas>
```
```javascript
const canvas = document.getElementById('canvas');
const ctx = canvas.getContext('2d');
const path = [
{ x: 50, y: 250 },
{ x: 100, y: 200 },
{ x: 150, y: 150 },
{ x: 200, y: 100 },
{ x: 250, y: 50 },
{ x: 300, y: 0 },
{ x: 350, y: 50 },
{ x: 400, y: 100 },
];
let index = 0;
let direction = 1;
function draw() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
ctx.beginPath();
ctx.moveTo(path[0].x, path[0].y);
for (let i = 1; i <= index; i++) {
const p1 = path[i - 1];
const p2 = path[i];
const dx = p2.x - p1.x;
const dy = p2.y - p1.y;
const cx = p1.x + dx / 2;
const cy = p1.y + dy / 2 - direction * Math.abs(dx) / 4;
ctx.quadraticCurveTo(cx, cy, p2.x, p2.y);
}
ctx.stroke();
const p1 = path[index - 1];
const p2 = path[index];
const dx = p2.x - p1.x;
const dy = p2.y - p1.y;
const angle = Math.atan2(dy, dx);
const arrowLength = 10;
const arrowWidth = 5;
const arrowX = p2.x - arrowLength * Math.cos(angle);
const arrowY = p2.y - arrowLength * Math.sin(angle);
ctx.beginPath();
ctx.moveTo(arrowX, arrowY);
ctx.lineTo(p2.x - arrowWidth * Math.sin(angle), p2.y - arrowWidth * Math.cos(angle));
ctx.lineTo(p2.x + arrowWidth * Math.sin(angle), p2.y + arrowWidth * Math.cos(angle));
ctx.fill();
index += direction;
if (index === path.length - 1 || index === 0) {
direction = -direction;
}
}
setInterval(draw, 50);
```
这段代码使用了一个数组 path 来存储股票路线的路径,使用 index 来表示当前绘制到的位置,使用 direction 来表示绘制的方向。在每次绘制时,先绘制路径,然后根据当前位置和方向计算箭头的位置和角度,最后绘制箭头。使用 setInterval 方法来定时更新绘制。
阅读全文
相关推荐
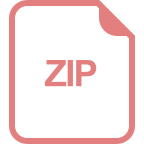
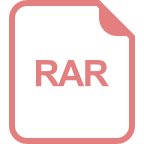
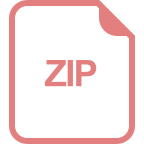















