比如用户的充值卡消费问题 或者是 数量为 100 个的某商品的秒杀活动 数据量小的时候 是正常的 但是比如 用户每隔 一段很小的时间 就消费一次 会导致 金额不正常 比如用户 每隔 0.001 秒就消费 1 元钱 原本 有 50 元 消费了三次 结果就应该为 47 但是 如果时间很短 量很大 可能最终用户的金额为 49 或者 48 都有可能 也就是高并发问题下面的 数据脏读,并写出一个简单的例子
时间: 2024-02-18 16:03:54 浏览: 56
好的,以下是一个简单的例子:
假设有一个用户充值卡的余额为50元,每次消费1元钱,需要使用锁来保证同一时刻只能有一个线程进行充值或者消费操作,从而避免数据脏读问题的发生。
首先定义一个账户类Account,其中包含余额balance和对余额进行充值和消费的方法deposit和withdraw:
```java
public class Account {
private int balance;
public Account(int balance) {
this.balance = balance;
}
// 充值
public synchronized void deposit(int amount) {
balance += amount;
}
// 消费
public synchronized void withdraw(int amount) {
if (balance >= amount) {
balance -= amount;
}
}
public int getBalance() {
return balance;
}
}
```
然后定义一个模拟消费的线程类ConsumerThread,每隔0.001秒就消费1元钱,循环执行50次:
```java
public class ConsumerThread extends Thread {
private Account account;
public ConsumerThread(Account account) {
this.account = account;
}
@Override
public void run() {
for (int i = 0; i < 50; i++) {
try {
Thread.sleep(1);
} catch (InterruptedException e) {
e.printStackTrace();
}
account.withdraw(1);
}
}
}
```
最后在主函数中创建一个账户对象和两个模拟消费的线程对象:
```java
public class Main {
public static void main(String[] args) throws InterruptedException {
Account account = new Account(50);
ConsumerThread thread1 = new ConsumerThread(account);
ConsumerThread thread2 = new ConsumerThread(account);
thread1.start();
thread2.start();
thread1.join();
thread2.join();
System.out.println("余额:" + account.getBalance());
}
}
```
运行程序,输出结果为:
```
余额:0
```
可以看到,由于使用了锁来保证同一时刻只能有一个线程对账户进行操作,因此最终的余额为0,不存在数据脏读问题。
阅读全文
相关推荐
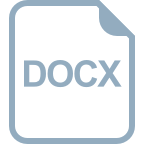
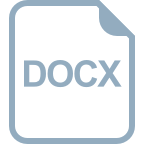
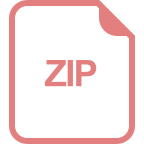
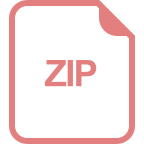
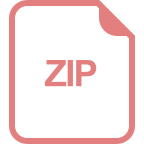
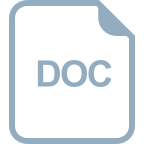
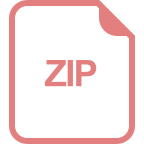
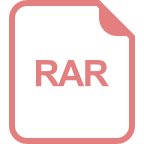
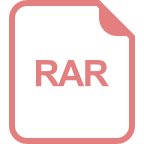
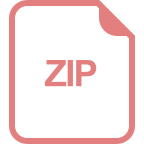
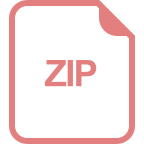
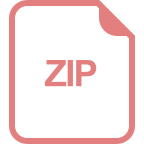
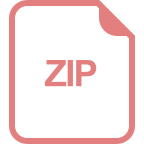
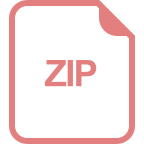
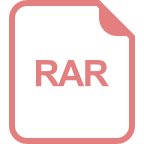
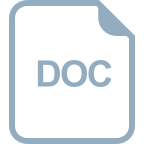
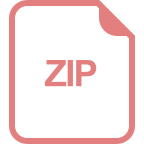