python程序(1)能接受至少2个网址的输入,并使用urllib模块从这些提供的网址上(2)下载20张以上包含猫、狗、熊猫等动物图片,(3)将其中是猫的图片保存在磁盘上(提示:可通过重命名图片),(4)并输出一共下载了多少图片、保存了多少张猫的图片、保存的图片位置的统计数据。
时间: 2024-06-08 08:09:51 浏览: 78
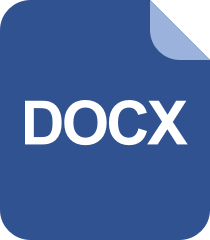
要使用Python爬取网站的照片,通常可以分为以下几个步骤:
以下是一个实现上述要求的 Python 程序:
```python
import urllib.request
import os
import re
# 输入网址列表
urls = ['http://www.example.com/1',
'http://www.example.com/2']
# 创建保存猫图片的文件夹
if not os.path.exists('cat_images'):
os.makedirs('cat_images')
# 计数器
total_count = 0
cat_count = 0
# 遍历每个网址
for url in urls:
# 下载网页内容
html = urllib.request.urlopen(url).read().decode('utf-8')
# 在网页内容中查找图片链接
img_links = re.findall(r'<img .*? src="(.*?\.(jpg|png))"', html)
# 遍历每个图片链接
for img_link in img_links:
# 下载图片
img_url = img_link[0]
img_filename = os.path.basename(img_url)
img_path = os.path.join('cat_images', img_filename)
urllib.request.urlretrieve(img_url, img_path)
total_count += 1
# 判断是否为猫图片并重命名保存
if 'cat' in img_filename:
cat_count += 1
cat_path = os.path.join('cat_images', f'cat_{cat_count}.jpg')
os.rename(img_path, cat_path)
# 输出统计数据
print(f'Total images downloaded: {total_count}')
print(f'Cat images saved: {cat_count}')
print(f'Images saved in: {os.path.abspath("cat_images")}')
```
其中使用了 `urllib.request` 模块下载网页内容和图片,使用了正则表达式匹配图片链接,并通过 `os` 模块操作文件和文件夹。程序会在当前目录下创建一个名为 `cat_images` 的文件夹,将所有下载的图片保存在其中。对于含有 `cat` 的图片,程序会将其重命名为 `cat_xxx.jpg` 的格式并保存,统计相关数据并输出。
阅读全文
相关推荐
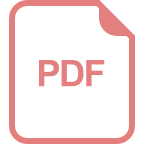
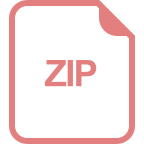






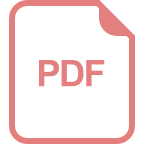
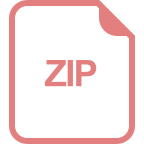
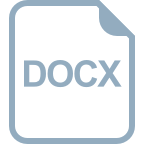
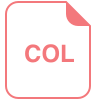
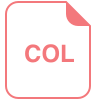
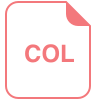
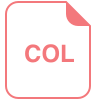
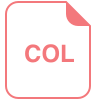
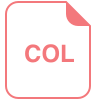