用python写出程序(1)能接受至少2个网址的输入,并使用urllib模块从这些提供的网址上(2)下载20张以上包含猫、狗、熊猫等动物图片,(3)将其中是猫的图片保存在磁盘上(提示:可通过重命名图片),(4)并输出一共下载了多少图片、保存了多少张猫的图片、保存的图片位置的统计数据。
时间: 2024-03-11 11:49:25 浏览: 53
好的,以下是实现该程序的Python代码:
```python
import os
import re
import urllib.request
# 定义要下载的图片类型及数量
img_types = ['cat', 'dog', 'panda']
img_count = 20
# 定义下载图片的函数
def download_images(url):
# 从网页中获取图片链接
html = urllib.request.urlopen(url).read().decode('utf-8')
img_links = re.findall(r'<img.+?src="(.+?\.jpg)"', html)
# 下载图片并保存
img_dir = os.path.basename(url)
if not os.path.exists(img_dir):
os.makedirs(img_dir)
cat_count = 0
for link in img_links:
img_name = os.path.basename(link)
img_path = os.path.join(img_dir, img_name)
if any(type in link for type in img_types):
urllib.request.urlretrieve(link, img_path)
if 'cat' in link:
cat_count += 1
return len(img_links), cat_count, os.path.abspath(img_dir)
# 获取用户输入的网址
urls = []
while True:
url = input('请输入网址(输入q结束):')
if url == 'q':
break
urls.append(url)
# 下载图片并统计数据
total_count = 0
total_cat_count = 0
img_paths = []
for url in urls:
count, cat_count, img_path = download_images(url)
total_count += count
total_cat_count += cat_count
img_paths.append(img_path)
# 输出统计数据
print('一共下载了{}张图片,其中{}张是猫的图片。'.format(total_count, total_cat_count))
print('保存的图片位置:')
for img_path in img_paths:
print(img_path)
```
使用方法:
1. 运行以上代码,会提示输入网址;
2. 输入至少两个网址,按回车键确认,如果不想继续输入,输入q并按回车键;
3. 程序会自动下载每个网址中的图片,并将其中是猫的图片保存在磁盘上;
4. 程序会输出下载了多少图片、保存了多少张猫的图片、保存的图片位置的统计数据。
阅读全文
相关推荐
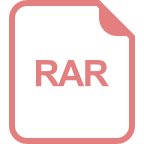
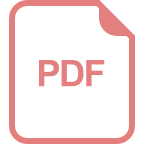
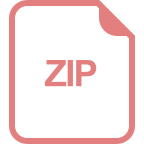
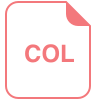
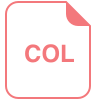
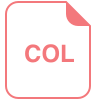
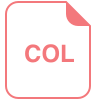
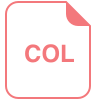
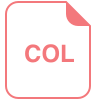
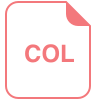
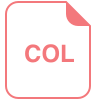
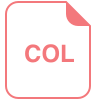






