button.ispressed
时间: 2023-11-27 20:50:13 浏览: 71
这是一个 Python 代码片段,用于检查按钮是否被按下。前提是已经定义了一个名为 `button` 的按钮对象,并且该对象具有 `ispressed` 属性。如果该属性值为 `True`,则表示按钮已被按下;否则,按钮未被按下。以下是一个示例代码片段:
```python
if button.ispressed:
print("Button is pressed!")
else:
print("Button is not pressed.")
```
相关问题
/** * An extension to JButton that binds the button action to a Driver object, * which must feature a method called buttonPressed() * * @author M. Allen */ import javax.swing.*; import java.awt.event.*; @SuppressWarnings( "serial" ) public class EventButton extends JButton implements ActionListener { private Calculator calculator; /** * pre: Driver d must be instantiated && must contain a method called * buttonPressed(). * post: This button can respond to action events and run the Driver method * buttonPressed(). * * @param d Driver with which this button communicates. */ public EventButton( Calculator c ) { super(); addActionListener( this ); calculator = c; } /** * post: If this button is pressed, the driver method buttonPressed() is * called to respond to the button presss event. * * @param e : ActionEvent from associated objects */ public void actionPerformed( ActionEvent e ) { calculator.buttonPressed( this ); } }
这段代码是一个继承自JButton的类EventButton,它绑定了按钮的操作到一个Calculator对象上,并要求该对象具有名为buttonPressed()的方法。当按钮被按下时,将调用Calculator对象的buttonPressed()方法来响应按钮按下事件。这段代码实现了基本的MVC模式中的控制器部分。
Write a simple GUI program with a button that responds to events from a mouse being pressed and released. The button should display: • the message “No action” when no action is taken on the mouse. • the message “Pressing down” when the mouse is pressed.
Here's a simple GUI program in Python using the tkinter module that displays a button and responds to mouse events:
```python
import tkinter as tk
class MouseButtonApp:
def __init__(self):
self.window = tk.Tk()
self.button = tk.Button(self.window, text="No action", command=self.no_action)
self.button.pack()
self.button.bind("<Button-1>", self.press_down)
self.button.bind("<ButtonRelease-1>", self.release_up)
def run(self):
self.window.mainloop()
def no_action(self):
self.button.configure(text="No action")
def press_down(self, event):
self.button.configure(text="Pressing down")
def release_up(self, event):
self.button.configure(text="No action")
app = MouseButtonApp()
app.run()
```
The `MouseButtonApp` class defines a window with a button, which initially displays "No action." The button is bound to two mouse events: `<Button-1>` for when the left mouse button is pressed down, and `<ButtonRelease-1>` for when it is released. When the button is pressed down, its text is changed to "Pressing down," and when it is released, it is changed back to "No action."
阅读全文
相关推荐
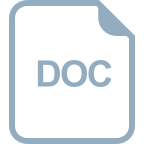
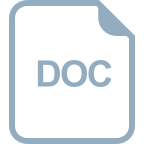
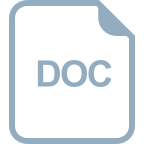
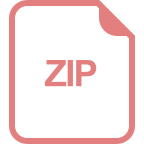
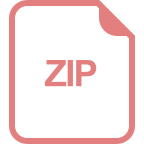












