英文注释这段代码#include "buct_hal.h" volatile int Button_Pressed_SW1, Button_Pressed_SW2; int main(void) { pinMode(GPIOF, 0, INPUT_PULLUP); pinMode(GPIOF, 4, INPUT_PULLUP); pinMode(GPIOF, 2, OUTPUT); pinMode(GPIOF, 1, OUTPUT); // Interrupt setup GPIOF_IS = GPIOF_IS & ~(1 << 0) & ~(1 << 4); GPIOF_IEV = GPIOF_IEV | (1 << 0) | (1 << 4); GPIOF_IM = GPIOF_IM | (1 << 0) | (1 << 4); SYS_EN0 = SYS_EN0 | (1 << 30); enable_interrupts(); while(1) { if (Button_Pressed_SW1) { Button_Pressed_SW1 = 0; redOn(); delay(1000000); redOff(); } if (Button_Pressed_SW2) { Button_Pressed_SW2 = 0; blueOn(); delay(1000000); blueOff(); } asm(" wfi "); // put the CPU to sleep. } return 0; } void GPIOF_Handler(void) { if (GPIOF_RIS & (1 << 0)) { Button_Pressed_SW2 = 1; GPIOF_ICR = (1 << 0); } if (GPIOF_RIS & (1 << 4)) { Button_Pressed_SW1 = 1; GPIOF_ICR = (1 << 4); } }
时间: 2024-04-01 20:34:54 浏览: 81
// This code includes the buct_hal.h library
#include "buct_hal.h"
// Define two global volatile variables to keep track of button presses
volatile int Button_Pressed_SW1, Button_Pressed_SW2;
// Main function
int main(void) {
// Set pin modes for buttons and LEDs
pinMode(GPIOF, 0, INPUT_PULLUP);
pinMode(GPIOF, 4, INPUT_PULLUP);
pinMode(GPIOF, 2, OUTPUT);
pinMode(GPIOF, 1, OUTPUT);
// Interrupt setup
// Configure interrupts for both buttons
GPIOF_IS = GPIOF_IS & ~(1 << 0) & ~(1 << 4);
// Set the interrupt event to occur on both rising and falling edges
GPIOF_IEV = GPIOF_IEV | (1 << 0) | (1 << 4);
// Enable interrupts for both buttons
GPIOF_IM = GPIOF_IM | (1 << 0) | (1 << 4);
// Enable the interrupt globally
SYS_EN0 = SYS_EN0 | (1 << 30);
// Enable interrupts in the CPU
enable_interrupts();
// Continuous loop to check for button presses
while(1)
{
// Check if SW1 button was pressed
if (Button_Pressed_SW1)
{
// Reset button pressed flag
Button_Pressed_SW1 = 0;
// Turn on the red LED
redOn();
// Delay for a short period of time
delay(1000000);
// Turn off the red LED
redOff();
}
// Check if SW2 button was pressed
if (Button_Pressed_SW2)
{
// Reset button pressed flag
Button_Pressed_SW2 = 0;
// Turn on the blue LED
blueOn();
// Delay for a short period of time
delay(1000000);
// Turn off the blue LED
blueOff();
}
// Put the CPU to sleep until the next interrupt occurs
asm(" wfi ");
}
// End of main function
return 0;
}
// Interrupt handler for GPIOF
void GPIOF_Handler(void) {
// Check if SW1 button was pressed
if (GPIOF_RIS & (1 << 0))
{
// Set the button pressed flag
Button_Pressed_SW2 = 1;
// Clear the interrupt flag
GPIOF_ICR = (1 << 0);
}
// Check if SW2 button was pressed
if (GPIOF_RIS & (1 << 4))
{
// Set the button pressed flag
Button_Pressed_SW1 = 1;
// Clear the interrupt flag
GPIOF_ICR = (1 << 4);
}
}
This code sets up interrupts for two buttons (SW1 and SW2) on GPIOF, and turns on/off LEDs based on the button presses. The main function continuously checks for button presses using a while loop, and puts the CPU to sleep until an interrupt occurs. The interrupt handler function (GPIOF_Handler) sets the appropriate button pressed flag and clears the interrupt flag. The code also uses the buct_hal.h library to configure pin modes and control the LEDs.
阅读全文
相关推荐
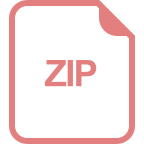
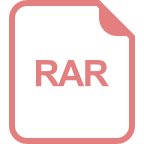
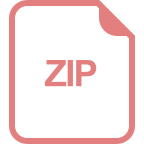
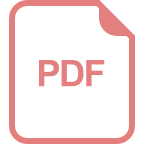
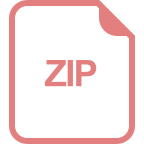
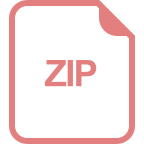
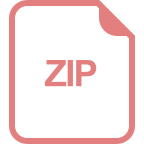
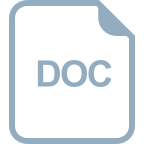










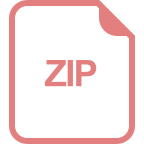