使用了Java 中的文件输出流 FileOutputStream 和对象输出流 ObjectOutputStream怎么把对象序列存到本地路径的map文件
时间: 2024-03-24 22:17:55 浏览: 64
可以按照以下步骤实现:
1. 创建一个Map对象,将需要存储的对象放入其中。
例如,假设需要存储一个名为person的对象,可以这样创建Map对象:
```
Map<String, Object> map = new HashMap<>();
map.put("person", person);
```
2. 创建一个文件输出流对象和一个对象输出流对象。
```
FileOutputStream fileOutputStream = new FileOutputStream("map.txt");
ObjectOutputStream objectOutputStream = new ObjectOutputStream(fileOutputStream);
```
3. 使用对象输出流对象将Map对象写入到文件中。
```
objectOutputStream.writeObject(map);
```
4. 关闭对象输出流和文件输出流。
```
objectOutputStream.close();
fileOutputStream.close();
```
完整的代码示例如下:
```
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.ObjectOutputStream;
import java.util.HashMap;
import java.util.Map;
public class Main {
public static void main(String[] args) {
// 创建一个Person对象
Person person = new Person("Tom", 20);
// 创建一个Map对象,将Person对象放入其中
Map<String, Object> map = new HashMap<>();
map.put("person", person);
try {
// 创建文件输出流和对象输出流
FileOutputStream fileOutputStream = new FileOutputStream("map.txt");
ObjectOutputStream objectOutputStream = new ObjectOutputStream(fileOutputStream);
// 将Map对象写入文件中
objectOutputStream.writeObject(map);
// 关闭输出流
objectOutputStream.close();
fileOutputStream.close();
} catch(IOException e) {
e.printStackTrace();
}
}
}
class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
```
执行完上述代码后,文件"map.txt"中将会存储一个包含了Person对象的Map对象。
阅读全文
相关推荐
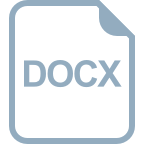
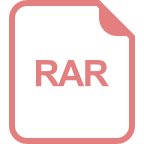
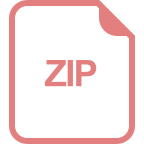

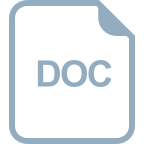
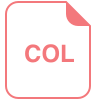
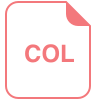
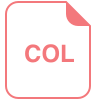
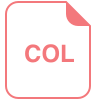
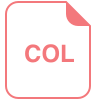
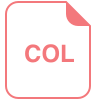
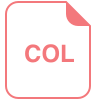
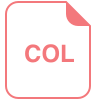
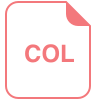
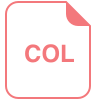
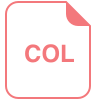


