package transpotion; import java.lang.reflect.InvocationTargetException; public class ComputeTime { public static void main(String[] args) { System.out.println("Mode of transportation is:"+args[0]); System.out.println("A="+args[1]); System.out.println("B="+args[2]); System.out.println("C="+args[3]); double a=Double.parseDouble(args[1]); double b=Double.parseDouble(args[2]); double c=Double.parseDouble(args[3]); double v,t; try { Common d=(Common) Class.forName("transpotion." +args[0]).getDeclaredConstructor().newInstance(); v=d.speed(a,b,c); t=1000/v; System.out.println("速度=:"+v+"km/h"); System.out.println("时间=:"+(float)t+"h"); } catch(ClassNotFoundException| InstantiationException | IllegalAccessException | NoSuchMethodException | InvocationTargetException e) { System.out.println("class not found"); } } } package transpotion; public interface Common { double speed(double a,double b,double c); } package transpotion; public class Car007 implements Common{ public double speed(double a, double b, double c) { if(c==0) { System.out.println("Divisor cannot be 0"); return 0; } else return a*b/c; } } package transpotion; public class Plane implements Common { public double speed(double a, double b, double c) { return a+b+c; } } package transpotion; public class Ship implements Common { public double speed(double a,double b,double c) { return a*b+a*c; } }可以为我讲解一下这段代码吗
时间: 2024-02-14 17:22:53 浏览: 25
这段代码是一个关于交通工具速度计算的示例程序,使用了Java语言实现。程序通过命令行输入三个参数,分别是交通工具名称、A参数、B参数和C参数,然后根据输入的交通工具名称使用反射机制创建对应的对象,调用该对象的速度计算方法,计算出速度和时间,并将结果输出。
其中,交通工具类实现了一个Common接口,该接口定义了一个速度计算的方法,不同的交通工具实现该接口方法的逻辑不同。Car007类实现了该接口,并重写了speed方法,用于计算汽车的速度;Plane类和Ship类同样实现了该接口,并分别重写了speed方法,用于计算飞机和船的速度。
需要注意的是,在使用反射机制创建对象时,需要通过Class.forName()方法传入完整的类名,包括包名。同时,对象的构造方法需要提供一个无参构造方法,否则将会抛出NoSuchMethodException异常。
相关问题
ClassFinal 使用后 Exception in thread "main" java.lang.reflect.InvocationTargetException
根据提供的引用内容,你在使用make px4_sitl jmavsim命令运行jMAVSim仿真时出现了错误提示Exception in thread "main" java.lang.reflect.InvocationTargetException。在将代码少量改动后,本地编译外加依赖打成jar包并部署到远程服务器时,运行出错,系统中查看报错信息: 出错原因: java.lang.reflect.InvocationTargetException。这个错误提示通常是由于Java反射机制调用方法时出现异常导致的。可能的原因是你的代码中存在一些错误,例如空指针异常或方法参数不正确等。你可以尝试以下方法来解决这个问题:
1.检查代码中是否存在语法错误或逻辑错误,特别是在使用反射机制时。
2.检查代码中是否存在空指针异常或方法参数不正确等错误。
3.检查你的代码是否与你的Java版本兼容。
4.检查你的代码是否正确地使用了依赖项。
5.尝试使用调试工具来查找问题所在。
java.lang.IllegalArgumentException: java.lang.reflect.InvocationTargetException
java.lang.IllegalArgumentException是Java中的一个异常类,表示传递给方法的参数不合法。而java.lang.reflect.InvocationTargetException是反射机制中的一个异常类,表示在调用方法时发生了异常。
当我们使用反射机制调用一个方法时,如果该方法本身抛出了异常,那么在使用反射调用时会将原始异常包装在InvocationTargetException中抛出。这样做是为了保持反射调用的一致性,无论是否使用反射调用,都可以通过捕获InvocationTargetException来处理异常。
常见的导致IllegalArgumentException和InvocationTargetException异常的原因包括:
- 传递给方法的参数类型不匹配或不合法,导致IllegalArgumentException异常。
- 被调用的方法本身抛出了异常,导致InvocationTargetException异常。
如果你遇到了这两个异常,可以通过查看异常堆栈信息来定位具体的问题所在,并根据具体情况进行处理。
相关推荐
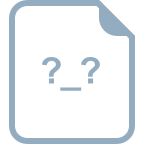
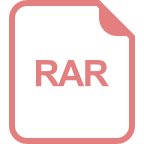
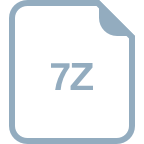













