python 实现二维码识别与坐标定位
时间: 2024-01-31 21:02:25 浏览: 353
要实现二维码的识别与坐标定位,可以使用 Python 中的 OpenCV 库和 pyzbar 库。这里提供一个简单的示例代码:
首先需要安装 pyzbar 库:
```python
pip install pyzbar
```
然后导入必要的库:
```python
import cv2
from pyzbar import pyzbar
```
接下来,读取图片并识别二维码:
```python
# 读取图片
image = cv2.imread('qrcode.jpg')
# 转换为灰度图像
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 找到二维码并识别
qrcodes = pyzbar.decode(gray)
```
此时,`qrcodes` 就是识别出的二维码列表,每个二维码包含了一些信息,比如二维码的类型、内容和位置等。可以通过遍历 `qrcodes` 列表来获取二维码的坐标:
```python
for qrcode in qrcodes:
# 获取二维码位置
(x, y, w, h) = qrcode.rect
# 在原图上绘制矩形
cv2.rectangle(image, (x, y), (x + w, y + h), (0, 255, 0), 2)
```
这里使用 `cv2.rectangle` 函数在原图上绘制矩形框,参数分别是原图、左上角坐标、右下角坐标、颜色和线宽。最后可以将绘制好的图片显示出来:
```python
# 显示图片
cv2.imshow('QRCodes', image)
cv2.waitKey(0)
```
完整代码如下:
```python
import cv2
from pyzbar import pyzbar
# 读取图片
image = cv2.imread('qrcode.jpg')
# 转换为灰度图像
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 找到二维码并识别
qrcodes = pyzbar.decode(gray)
# 遍历二维码并绘制矩形框
for qrcode in qrcodes:
# 获取二维码位置
(x, y, w, h) = qrcode.rect
# 在原图上绘制矩形
cv2.rectangle(image, (x, y), (x + w, y + h), (0, 255, 0), 2)
# 显示图片
cv2.imshow('QRCodes', image)
cv2.waitKey(0)
```
运行代码后,就可以看到识别出的二维码并且已经绘制好了矩形框。
阅读全文
相关推荐
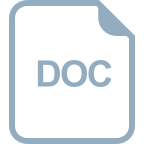
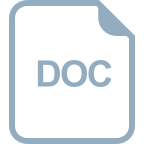
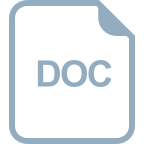
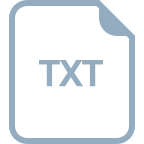
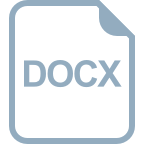






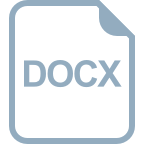
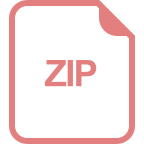
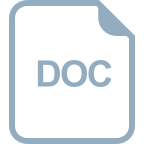
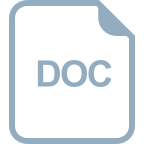
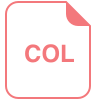
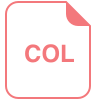
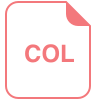
