Collectors.summingInt()
时间: 2023-06-23 15:51:25 浏览: 113
`Collectors.summingInt()` 是Java 8中提供的一个收集器(Collector),它用于将流中元素的一个 `int` 属性进行求和操作,并返回一个 `int` 值作为结果。它的使用方式如下:
```
int sum = list.stream()
.collect(Collectors.summingInt(MyClass::getNumber));
```
这里的 `MyClass` 是一个自定义类,在类中定义了一个 `int` 属性 `number`,并提供了一个公共的 `getNumber()` 方法用于获取该属性的值。`Collectors.summingInt()` 方法将会对流中所有元素的 `number` 属性进行求和操作,并返回一个 `int` 值作为结果。
相关问题
Collectors.summingInt
Collectors.summingInt is a static method in the Java Stream API that returns a collector which calculates the sum of a specified integer attribute in a stream of objects. It is commonly used in conjunction with the Stream.collect method.
Here's an example usage:
```
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class Main {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
int sum = numbers.stream()
.collect(Collectors.summingInt(num -> num));
System.out.println("Sum: " + sum);
}
}
```
In this example, we have a list of integers `numbers`. We use the `stream()` method to create a stream from the list and then apply the `collect` method with `Collectors.summingInt` as the collector. The lambda expression `num -> num` specifies the integer attribute we want to sum. Finally, we print the sum which is the result of the `Collectors.summingInt` operation.
Collectors.summingInt 为null 时候取0
`Collectors.summingInt` 是 Java Stream API 中的一个收集器,用于对整数类型的元素进行求和操作。当你尝试使用这个方法处理一个空集合(null 或者长度为 0 的集合),它默认会返回 `Integer` 类型的零值,也就是 0。
例如:
```java
List<Integer> numbers = null;
OptionalLong sum = numbers.stream()
.collect(Collectors.summingInt(Integer::intValue));
if (sum.isPresent()) {
int total = sum.getAsInt(); // 如果numbers为空,total将为0
} else {
System.out.println("Numbers list is empty or null");
}
```
如果 `numbers` 为 `null`,上述代码会直接打印出 "Numbers list is empty or null",而不会抛出异常,因为 `sum` 是一个 `OptionalLong`,它的 `isPresent()` 方法可以检查是否存在非空结果。
阅读全文
相关推荐
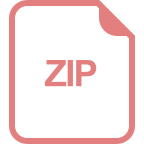
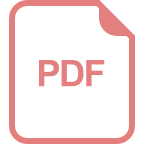
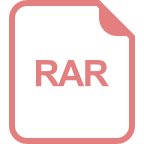






