#include<iostream> #include<cstring> using namespace std; class Person { private:char name[40]; char sex[3]; int age; public: Person() { strcpy(name,"Unknown"); strcpy(sex,"No"); age=0; cout<<"Function #1 is called!"<<endl; } Person(const char na[40], const char se[3], int a) { strcpy(name, na); strcpy(sex, se); age = a; cout << "Function #2 is called!" << endl; } Person(const Person& p) { strcpy(name,p.name); strcpy(sex,p.sex); age=p.age; } ~Person(){}; void Show(); void Show2(); }; void Person::Show() { cout<<"NAME:"<<name<<" "<<"SEX:"<<sex<<" "<<"AGE:"<<age<<endl; cout<<"Function #3 is called!"<<endl; } void Person::Show2() { cout<<"NAME:"<<name<<" "<<"SEX:"<<sex<<" "<<"AGE:"<<age<<" "; } class Teacher:public Person { private:char title[40]; int pay; public: Teacher():Person() { strcpy(title,"NONE"); pay=0; cout<<"Function #4 is called!"<<endl; } Teacher(char *na,char *se,int a,char *t,int p):Person(na,se,a) { strcpy(title,t); pay=p; cout<<"Function #5 is called!"<<endl; } Teacher(const Person& p,char *t,int pa):Person(p) { strcpy(title,t); pay=pa; cout<<"Function #6 is called!"<<endl; } Teacher(char *t,int p):Person() { strcpy(title,t); pay=p; cout<<"Function #7 is called!"<<endl; } Teacher(const Teacher& t):Person(t) { strcpy(title,t.title); pay=t.pay; } Teacher(const Person& p):Person(p){ strcpy(title,"NONE"); pay=0; cout<<"Function #8 is called!"<<endl; } ~Teacher(){}; void Show(); }; void Teacher::Show() { Person::Show2(); cout<<""<<"TLTLE:"<<title<<" "<<"PAY:"<<pay<<endl; cout<<"Function #9 is called!"<<endl; } int main() { #ifdef _CRT_SECURE_NO_WARNINGS freopen("./in.txt", "r", stdin); freopen("./out.txt", "w", stdout); #endif char name[40],sex[3],title[40]; int age,pay; cin>>name>>sex>>age>>title>>pay; Person p1; cout<<"Person #1:"; p1.Show(); Person p2(name,sex,age); cout<<"Person #2:"; p2.Show(); Teacher t1; cout<<"Teacher #1:"; t1.Show(); Teacher t2(name,sex,age,title,pay); cout<<"Teacher #2:"; t2.Show(); Teacher t3(p2,title,pay); cout<<"Teacher #3:"; t3.Show(); Teacher t4(title,pay); cout<<"Teacher #4:"; t4.Show(); Teacher t5(p2); cout<<"Teacher #5:"; t5.Show(); return 0; },优化这段代码
时间: 2024-03-03 15:48:51 浏览: 16
以下是对代码的优化:
- 将字符串类型的参数改为 const char*,避免字符串拷贝和内存浪费。
- 在类中使用成员初始化列表,避免在构造函数中使用 strcpy() 进行字符串拷贝。
- 将类中的 Show() 函数改为 const 成员函数,避免对类成员的修改。
- 使用 nullptr 替代 NULL。
- 将 #ifdef _CRT_SECURE_NO_WARNINGS 放在文件开头。
以下是优化后的代码:
```cpp
#include<iostream>
#include<cstring>
using namespace std;
class Person {
private:
char name[40] = "Unknown";
char sex[3] = "No";
int age = 0;
public:
Person() {
cout << "Function #1 is called!" << endl;
}
Person(const char* na, const char* se, int a)
: age(a) {
strcpy(name, na);
strcpy(sex, se);
cout << "Function #2 is called!" << endl;
}
Person(const Person& p) {
strcpy(name, p.name);
strcpy(sex, p.sex);
age = p.age;
}
void Show() const {
cout << "NAME:" << name << " " << "SEX:" << sex << " " << "AGE:" << age << endl;
cout << "Function #3 is called!" << endl;
}
void Show2() const {
cout << "NAME:" << name << " " << "SEX:" << sex << " " << "AGE:" << age << " ";
}
};
class Teacher : public Person {
private:
char title[40] = "NONE";
int pay = 0;
public:
Teacher() : Person() {
cout << "Function #4 is called!" << endl;
}
Teacher(const char* na, const char* se, int a, const char* t, int p)
: Person(na, se, a), pay(p) {
strcpy(title, t);
cout << "Function #5 is called!" << endl;
}
Teacher(const Person& p, const char* t, int pa)
: Person(p), pay(pa) {
strcpy(title, t);
cout << "Function #6 is called!" << endl;
}
Teacher(const char* t, int p) : Person() {
strcpy(title, t);
pay = p;
cout << "Function #7 is called!" << endl;
}
Teacher(const Teacher& t) : Person(t) {
strcpy(title, t.title);
pay = t.pay;
}
Teacher(const Person& p) : Person(p) {
cout << "Function #8 is called!" << endl;
}
void Show() const {
Person::Show2();
cout << "" << "TLTLE:" << title << " " << "PAY:" << pay << endl;
cout << "Function #9 is called!" << endl;
}
};
int main() {
#ifdef _CRT_SECURE_NO_WARNINGS
freopen("./in.txt", "r", stdin);
freopen("./out.txt", "w", stdout);
#endif
char name[40], sex[3], title[40];
int age, pay;
cin >> name >> sex >> age >> title >> pay;
Person p1;
cout << "Person #1:";
p1.Show();
Person p2(name, sex, age);
cout << "Person #2:";
p2.Show();
Teacher t1;
cout << "Teacher #1:";
t1.Show();
Teacher t2(name, sex, age, title, pay);
cout << "Teacher #2:";
t2.Show();
Teacher t3(p2, title, pay);
cout << "Teacher #3:";
t3.Show();
Teacher t4(title, pay);
cout << "Teacher #4:";
t4.Show();
Teacher t5(p2);
cout << "Teacher #5:";
t5.Show();
return 0;
}
```
相关推荐
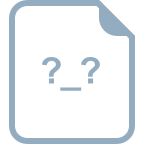















