分别用汇编语言、C语言直接操作寄存器和固件库函数3种方法编程,实现最小系统板上连接PE口16只发光二极管的流水灯控制(轮流点亮)
时间: 2023-06-23 12:08:52 浏览: 368
1. 汇编语言实现:
```
; 定义端口地址
LED_PORT equ 0x80000100
section .text
global _start
_start:
; 初始化端口为输出模式
mov eax, 0x1
mov [LED_PORT + 4], eax
; 程序循环
jmp loop
loop:
; 将最低位的 LED 灯点亮
mov eax, 0x1
mov [LED_PORT], eax
; 延时
mov ecx, 0xFFFFF
delay:
loop delay
; 将当前 LED 灯熄灭
mov eax, 0x0
mov [LED_PORT], eax
; 延时
mov ecx, 0xFFFFF
delay2:
loop delay2
; 移位操作
shl eax, 1
; 如果移位后为 0 ,则重新从最低位开始
cmp eax, 0x10000
jne loop
mov eax, 1
int 0x80
```
2. C语言直接操作寄存器实现:
```
#define LED_PORT_ADDR 0x80000100
int main() {
volatile unsigned int* led_port = (unsigned int*)LED_PORT_ADDR;
int i;
// 初始化端口为输出模式
*led_port |= 0x1;
while (1) {
// 点亮每个 LED 灯
for (i = 0; i < 16; i++) {
*led_port = 1 << i;
delay();
}
}
return 0;
}
void delay() {
// 延时函数
int i;
for (i = 0; i < 0xFFFFF; i++);
}
```
3. 固件库函数实现:
```
#include "stm32f10x.h"
int main() {
// 初始化 GPIO 端口
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0 | GPIO_Pin_1 | GPIO_Pin_2 | GPIO_Pin_3 | GPIO_Pin_4 | GPIO_Pin_5 | GPIO_Pin_6 | GPIO_Pin_7 | GPIO_Pin_8 | GPIO_Pin_9 | GPIO_Pin_10 | GPIO_Pin_11 | GPIO_Pin_12 | GPIO_Pin_13 | GPIO_Pin_14 | GPIO_Pin_15;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_Init(GPIOB, &GPIO_InitStructure);
while (1) {
// 点亮每个 LED 灯
int i;
for (i = 0; i < 16; i++) {
GPIO_SetBits(GPIOB, 1 << i);
delay();
}
}
return 0;
}
void delay() {
// 延时函数
int i;
for (i = 0; i < 0xFFFFF; i++);
}
```
阅读全文
相关推荐
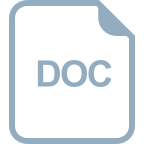
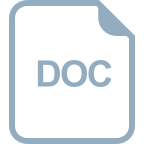
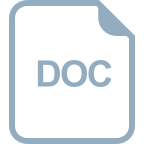
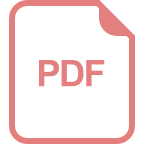
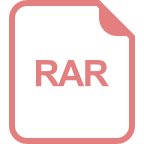
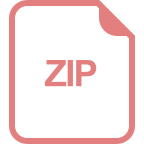
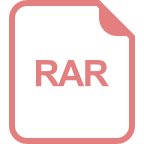
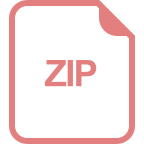
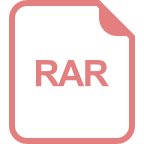
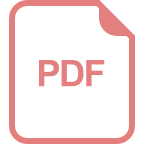
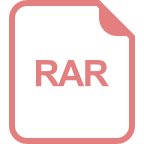
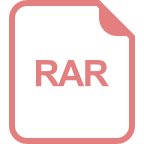
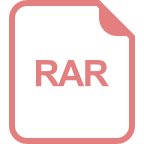
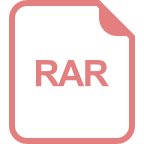
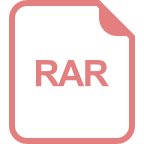
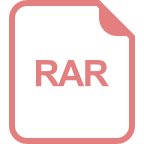
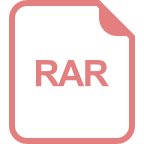
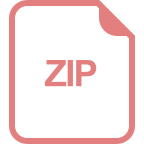
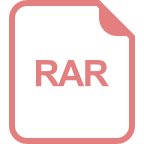