书写使用springboot和Vue建立学生选课系统的代码
时间: 2024-01-08 17:04:14 浏览: 29
这个需求涉及到前后端的开发,需要分别建立Spring Boot后端和Vue前端的工程。下面给出一个简单的示例代码:
Spring Boot后端:
1. 创建Spring Boot工程
2. 添加依赖
```xml
<!--Spring Boot Web-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!--Spring Boot Data JPA-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<!--MySQL驱动-->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
```
3. 配置数据库连接
```properties
spring.datasource.url=jdbc:mysql://localhost:3306/db_example
spring.datasource.username=springuser
spring.datasource.password=ThePassword
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
```
4. 定义实体类
```java
@Entity
@Table(name="student")
public class Student {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Integer id;
private String name;
private String gender;
private String major;
private Integer grade;
// Getters and Setters
}
```
5. 定义Repository接口
```java
@Repository
public interface StudentRepository extends JpaRepository<Student, Integer> {
}
```
6. 定义Controller
```java
@RestController
@RequestMapping("/api")
public class StudentController {
@Autowired
private StudentRepository studentRepository;
@GetMapping("/students")
public List<Student> getStudents() {
return studentRepository.findAll();
}
@PostMapping("/students")
public Student addStudent(@RequestBody Student student) {
return studentRepository.save(student);
}
@DeleteMapping("/students/{id}")
public void deleteStudent(@PathVariable Integer id) {
studentRepository.deleteById(id);
}
}
```
7. 运行工程
Vue前端:
1. 创建Vue工程
2. 安装axios
```bash
npm install axios --save
```
3. 编写组件
```vue
<template>
<div class="container">
<h2>学生选课系统</h2>
<div class="form-group">
<label for="name">姓名:</label>
<input type="text" class="form-control" v-model="student.name" id="name">
</div>
<div class="form-group">
<label for="gender">性别:</label>
<input type="text" class="form-control" v-model="student.gender" id="gender">
</div>
<div class="form-group">
<label for="major">专业:</label>
<input type="text" class="form-control" v-model="student.major" id="major">
</div>
<div class="form-group">
<label for="grade">年级:</label>
<input type="number" class="form-control" v-model="student.grade" id="grade">
</div>
<button class="btn btn-primary" @click="addStudent">添加学生</button>
<table class="table mt-3">
<thead>
<tr>
<th>编号</th>
<th>姓名</th>
<th>性别</th>
<th>专业</th>
<th>年级</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr v-for="student in students" :key="student.id">
<td>{{student.id}}</td>
<td>{{student.name}}</td>
<td>{{student.gender}}</td>
<td>{{student.major}}</td>
<td>{{student.grade}}</td>
<td>
<button class="btn btn-danger" @click="deleteStudent(student.id)">删除</button>
</td>
</tr>
</tbody>
</table>
</div>
</template>
<script>
import axios from 'axios';
export default {
data() {
return {
student: {
name: '',
gender: '',
major: '',
grade: ''
},
students: []
}
},
mounted() {
this.getStudents();
},
methods: {
getStudents() {
axios.get('/api/students')
.then(response => {
this.students = response.data;
});
},
addStudent() {
axios.post('/api/students', this.student)
.then(response => {
this.students.push(response.data);
this.student.name = '';
this.student.gender = '';
this.student.major = '';
this.student.grade = '';
});
},
deleteStudent(id) {
axios.delete(`/api/students/${id}`)
.then(() => {
this.getStudents();
});
}
}
};
</script>
```
4. 运行工程
以上是一个简单的示例代码,具体实现需要根据实际需求进行调整。
相关推荐
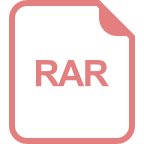
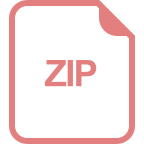














