java class文件加密的代码实现
时间: 2023-11-13 16:06:33 浏览: 42
Java Class 文件加密可以通过以下步骤实现:
1. 读取源 Class 文件
2. 对 Class 文件进行加密处理
3. 将加密后的 Class 文件写入到目标文件中
以下是一个简单的 Java Class 文件加密的代码实现示例:
```java
import java.io.*;
public class ClassFileEncryptor {
public static void main(String[] args) throws Exception {
if (args.length < 2) {
System.err.println("Usage: java ClassFileEncryptor <source> <target>");
System.exit(1);
}
String sourceFile = args[0];
String targetFile = args[1];
byte[] classBytes = readClassFile(sourceFile);
byte[] encryptedBytes = encrypt(classBytes);
writeEncryptedClassFile(encryptedBytes, targetFile);
}
private static byte[] readClassFile(String fileName) throws Exception {
InputStream is = new FileInputStream(fileName);
ByteArrayOutputStream buffer = new ByteArrayOutputStream();
int nRead;
byte[] data = new byte[1024];
while ((nRead = is.read(data, 0, data.length)) != -1) {
buffer.write(data, 0, nRead);
}
buffer.flush();
return buffer.toByteArray();
}
private static byte[] encrypt(byte[] input) {
// perform encryption operation here
byte[] output = new byte[input.length];
for (int i = 0; i < input.length; i++) {
output[i] = (byte) (input[i] ^ 0xff);
}
return output;
}
private static void writeEncryptedClassFile(byte[] encryptedBytes, String targetFile) throws Exception {
OutputStream os = new FileOutputStream(targetFile);
os.write(encryptedBytes);
os.flush();
os.close();
}
}
```
这个示例程序包含了三个方法:
1. `readClassFile` 方法,用于读取源 Class 文件的内容。
2. `encrypt` 方法,用于加密 Class 文件内容。
3. `writeEncryptedClassFile` 方法,用于将加密后的 Class 文件写入目标文件。
在这个示例中,加密操作是通过对每个字节执行按位异或操作实现的。在实际应用中,可以使用更加安全的加密算法来进行加密操作。
相关推荐
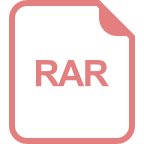
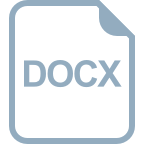
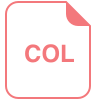
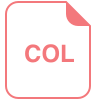
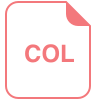
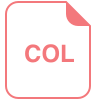
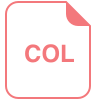









