如何使用DIBuilder和setDebugLoc为自定义LLVM pass添加调试信息,给出一个示例
时间: 2023-11-28 10:47:21 浏览: 77
在 LLVM IR 中添加调试信息是非常重要的,因为它可以帮助我们更好地理解程序的执行过程。在 LLVM 中,可以使用 DIBuilder 和 setDebugLoc 函数来为自定义 Pass 添加调试信息。
以下是一个简单的示例,演示如何使用 DIBuilder 和 setDebugLoc 函数为 LLVM IR 中的指令添加调试信息:
```cpp
#include "llvm/IR/DebugInfo.h"
#include "llvm/IR/DIBuilder.h"
#include "llvm/IR/DebugLoc.h"
#include "llvm/IR/Function.h"
#include "llvm/IR/Instructions.h"
#include "llvm/Pass.h"
#include "llvm/Support/raw_ostream.h"
using namespace llvm;
namespace {
struct MyDebugPass : public FunctionPass {
static char ID;
MyDebugPass() : FunctionPass(ID) {}
bool runOnFunction(Function &F) override {
LLVMContext &C = F.getContext();
// Create the DIBuilder
DIBuilder DIB(C);
// Create a DebugLoc for line 1 of the function
DebugLoc loc = DebugLoc::get(1, 0, DIB.createFile("test.ll", "."));
// Loop over all instructions in the Function
for (auto &BB : F) {
for (auto &I : BB) {
// Add a DebugLoc to the instruction
I.setDebugLoc(loc);
// Print the instruction with its DebugLoc
errs() << I << "\n";
}
}
return false;
}
};
}
char MyDebugPass::ID = 0;
static RegisterPass<MyDebugPass> X("my-debug-pass", "My Debug Pass");
```
在上面的示例中,我们首先使用 DIBuilder 创建一个 DebugLoc 对象,该对象表示源代码中的行号和文件名。然后,我们遍历函数中的所有指令,并使用 setDebugLoc 函数将 DebugLoc 添加到每个指令中。最后,我们打印每个指令及其 DebugLoc。
在运行此 Pass 时,它会输出带有调试信息的每个指令,例如:
```
%0 = alloca i32, align 4, !dbg !5
```
其中 `!dbg` 是 LLVM 中用于表示调试信息的元数据标记。
阅读全文
相关推荐
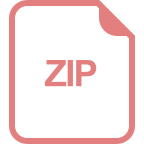
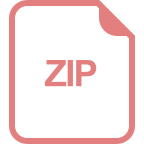
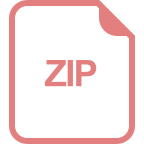
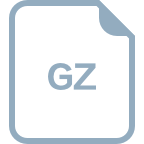
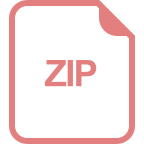
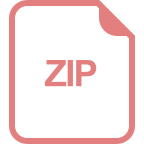
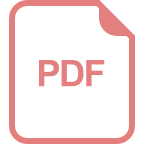
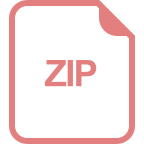
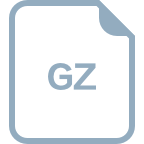
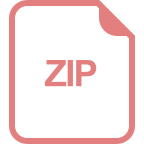
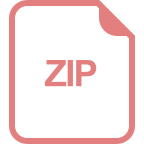
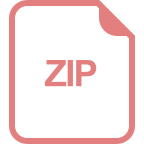
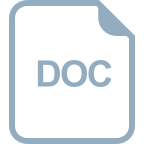
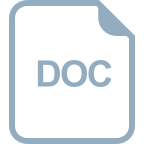



