在LLVM自定义的pass中,向IR中插入一个函数,该函数以llvm::instruction::getdebugloc()->getfilename()为输入参数
时间: 2023-12-06 11:45:37 浏览: 33
可以参考下面的代码示例:
```c++
#include "llvm/IR/LLVMContext.h"
#include "llvm/IR/Module.h"
#include "llvm/IR/Function.h"
#include "llvm/IR/BasicBlock.h"
#include "llvm/IR/IRBuilder.h"
#include "llvm/IR/DebugInfoMetadata.h"
#include "llvm/IR/DIBuilder.h"
using namespace llvm;
namespace {
class MyPass : public FunctionPass {
public:
static char ID;
MyPass() : FunctionPass(ID) {}
bool runOnFunction(Function &F) override {
LLVMContext &Ctx = F.getContext();
Module *M = F.getParent();
// Get the DebugInfoFinder from the module
DebugInfoFinder &DIFinder = M->getDebugInfoFinder();
// Get the debug location of the first instruction in the function
Instruction *FirstInst = &*F.getEntryBlock().begin();
DebugLoc DL = FirstInst->getDebugLoc();
if (!DL) {
// No debug location available
return false;
}
// Get the file name from the debug location
StringRef FileName = DL->getFilename();
// Create the new function
Type *RetTy = Type::getVoidTy(Ctx);
Type *ArgTy = Type::getInt8PtrTy(Ctx);
FunctionType *FuncTy = FunctionType::get(RetTy, ArgTy, false);
Function *NewFunc = Function::Create(FuncTy, GlobalValue::ExternalLinkage,
"myfunc", M);
// Create the entry basic block of the new function
BasicBlock *BB = BasicBlock::Create(Ctx, "entry", NewFunc);
// Insert a return instruction at the end of the basic block
IRBuilder<> Builder(BB);
Builder.CreateRetVoid();
// Set the debug location for the return instruction
DIBuilder DIB(*M);
DILocation *DIL = DIB.createFile(FileName, ".");
Builder.SetCurrentDebugLocation(DIL->getLine(), 0, DIL);
// Return true to indicate that the function was modified
return true;
}
};
char MyPass::ID = 0;
static RegisterPass<MyPass> X("mypass", "My custom pass", false, false);
} // end anonymous namespace
```
这个pass会遍历函数中的所有基本块,并在每个基本块的开头插入一个新函数。新函数的参数类型是`i8*`,返回类型是`void`。新函数不做任何操作,只是简单地返回。在创建新函数之后,我们使用`DIBuilder`类创建了一个新的调试位置,然后将该位置与新函数的返回指令相关联。
相关推荐
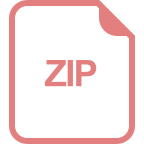
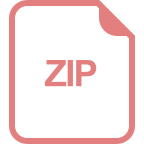














