c++ 用<Windows.h> 遍历路径文件夹中所有文件夹和文件 复制到指认的路径中
时间: 2024-05-10 07:20:46 浏览: 170
以下是使用<Windows.h>实现遍历路径文件夹中所有文件夹和文件复制到指定路径中的示例代码:
```c
#include <Windows.h>
#include <stdio.h>
#include <string.h>
void copyDirectory(const char* srcPath, const char* destPath);
int main()
{
const char* srcPath = "C:\\Path\\To\\Source\\Folder";
const char* destPath = "C:\\Path\\To\\Destination\\Folder";
copyDirectory(srcPath, destPath);
return 0;
}
void copyDirectory(const char* srcPath, const char* destPath)
{
char src[MAX_PATH], dest[MAX_PATH];
WIN32_FIND_DATA findData;
HANDLE hFind;
// Create the destination directory if it doesn't exist
CreateDirectory(destPath, NULL);
// Append wildcard to the source path
strcpy(src, srcPath);
strcat(src, "\\*");
// Find the first file in the source directory
hFind = FindFirstFile(src, &findData);
if (hFind == INVALID_HANDLE_VALUE)
{
printf("Error: Invalid handle value.\n");
return;
}
do
{
// Skip . and .. directories
if (strcmp(findData.cFileName, ".") == 0 || strcmp(findData.cFileName, "..") == 0)
continue;
// Build the full path for the source and destination files
sprintf(src, "%s\\%s", srcPath, findData.cFileName);
sprintf(dest, "%s\\%s", destPath, findData.cFileName);
// If the file is a directory, recursively copy the directory
if (findData.dwFileAttributes & FILE_ATTRIBUTE_DIRECTORY)
copyDirectory(src, dest);
else // Otherwise, copy the file
CopyFile(src, dest, FALSE);
} while (FindNextFile(hFind, &findData));
// Close the handle and return
FindClose(hFind);
}
```
该代码通过递归遍历源目录中的所有文件夹和文件,并将它们复制到目标目录中。其中,使用了Win32 API中的一些函数,如FindFirstFile、CreateDirectory和CopyFile等。需要注意的是,如果复制的文件已经存在于目标目录中,则会覆盖原有文件。
阅读全文
相关推荐
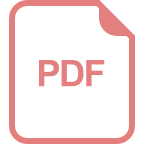

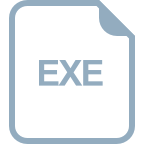
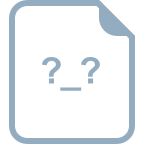
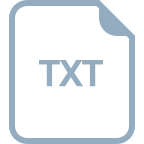
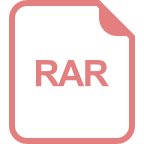


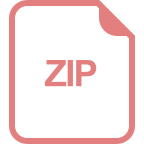
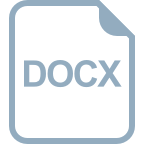
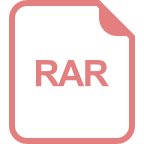
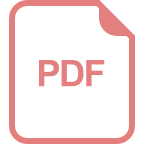


