c++ 代码中 HANDLE 遍历指定路径下所有 文件夹以及所有文件,还有子文件夹中所有文件夹及所有文件并把所有遍历到的文件夹以及文件复制到指定的路径中
时间: 2023-09-19 07:07:27 浏览: 169
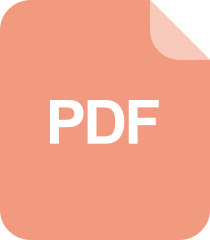
C++各种遍历文件夹代码.pdf
以下是遍历指定路径下所有文件夹以及所有文件的 c 代码:
```c
#include <windows.h>
#include <stdio.h>
#include <stdbool.h>
void TraverseDirectory(char* path, char* destPath)
{
char searchPath[MAX_PATH];
WIN32_FIND_DATA findData;
HANDLE hFind;
// 遍历指定路径下的文件夹
sprintf(searchPath, "%s\\*", path);
hFind = FindFirstFile(searchPath, &findData);
if (hFind != INVALID_HANDLE_VALUE)
{
do
{
if (findData.dwFileAttributes & FILE_ATTRIBUTE_DIRECTORY)
{
// 如果是文件夹且不是"."或".."目录,则递归遍历该文件夹中的文件
if (strcmp(findData.cFileName, ".") != 0 && strcmp(findData.cFileName, "..") != 0)
{
char subPath[MAX_PATH];
sprintf(subPath, "%s\\%s", path, findData.cFileName);
TraverseDirectory(subPath, destPath);
}
}
else
{
// 如果是文件,则复制到指定路径中
char srcPath[MAX_PATH];
char destFilePath[MAX_PATH];
sprintf(srcPath, "%s\\%s", path, findData.cFileName);
sprintf(destFilePath, "%s\\%s", destPath, findData.cFileName);
CopyFile(srcPath, destFilePath, false);
}
} while (FindNextFile(hFind, &findData));
FindClose(hFind);
}
}
```
在上面的代码中,我们通过 `FindFirstFile` 和 `FindNextFile` 函数遍历指定路径下的文件夹和文件。如果遍历到的是文件夹,则递归遍历该文件夹中的文件;如果遍历到的是文件,则复制到指定路径中。
下面是调用上述函数的代码:
```c
int main()
{
char path[MAX_PATH] = "C:\\test";
char destPath[MAX_PATH] = "D:\\backup";
TraverseDirectory(path, destPath);
return 0;
}
```
在上面的代码中,我们将需要遍历的路径和要备份到的路径传递给 `TraverseDirectory` 函数,该函数会遍历指定路径下的所有文件夹和文件,并将其复制到指定的备份路径中。
阅读全文
相关推荐
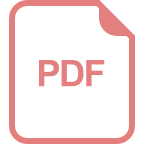

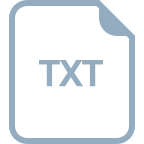
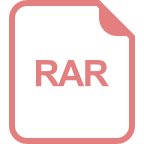
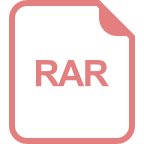



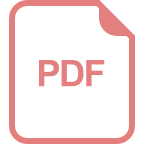
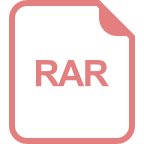
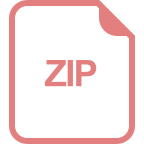
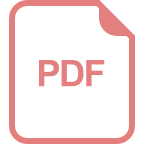
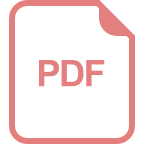
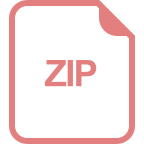
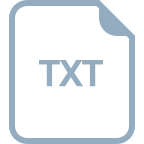
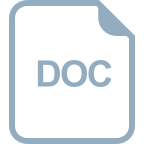
