python删除字符串中的反斜杠
时间: 2023-09-10 16:05:41 浏览: 218
### 回答1:
在 Python 中,可以使用字符串的 `replace()` 方法来删除字符串中的反斜杠。例如:
```
string = "hello\\world"
string = string.replace("\\", "")
print(string) # 输出 "helloworld"
```
也可以使用字符串的 `strip()` 方法来删除字符串两端的反斜杠:
```
string = "\\hello\\world\\"
string = string.strip("\\")
print(string) # 输出 "hello\\world"
```
注意,如果要在字符串中删除所有的反斜杠,则需要使用循环,例如:
```
string = "hello\\world\\"
while "\\" in string:
string = string.replace("\\", "")
print(string) # 输出 "helloworld"
```
### 回答2:
在Python中删除字符串中的反斜杠可以使用字符串的replace()方法。replace()方法用于将字符串中的指定子字符串替换为新的字符串,并返回替换后的字符串。具体实现如下:
```python
# 原始字符串
string = "Hello\\ World\\"
# 使用replace()方法删除反斜杠
new_string = string.replace("\\", "")
# 输出删除反斜杠后的字符串
print(new_string)
```
以上代码中,我们定义了一个原始字符串string,其中包含了反斜杠。然后使用replace()方法将反斜杠替换为空字符串""。最后,将替换后的字符串打印出来即可。
注意,由于Python中的反斜杠是一个特殊字符,表示转义字符,所以在字符串中使用反斜杠时需要使用双反斜杠"\\\\"来表示一个普通的反斜杠。
### 回答3:
在Python中,我们可以使用字符串的replace()方法来删除反斜杠。replace()方法接受两个参数,第一个参数是要替换的子字符串,第二个参数是用于替换的字符串。由于反斜杠是一个转义字符,所以我们需要在替换参数中使用两个反斜杠来表示一个反斜杠。以下是一个示例代码:
```python
string = "Hello\\World!"
new_string = string.replace("\\", "")
print(new_string)
```
运行以上代码,输出的结果将不包含反斜杠,也就是"HelloWorld!"。
在这个示例中,我们首先定义了一个包含反斜杠的字符串"Hello\\World!"。然后使用replace()方法,将字符串中的反斜杠替换为空字符串。最后打印新的字符串,即删除了反斜杠的结果。
需要注意的是,由于反斜杠是一个特殊字符,如果我们想要在字符串中保留一个反斜杠,就需要使用两个反斜杠进行转义,例如"\\\\"。
相关推荐
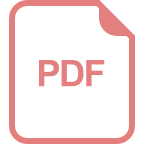
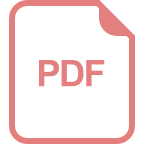
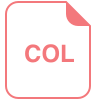
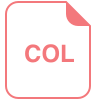
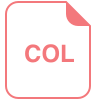
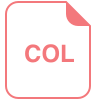









