保序加密是什么?有哪几种?分别怎么用C语言实现
时间: 2023-06-11 09:05:56 浏览: 332
保序加密(Order-Preserving Encryption,OPE)是一种加密方式,它可以将明文转换为密文,同时保持明文的大小关系不变。也就是说,如果明文 $m_1 < m_2$,那么密文 $c_1 < c_2$。这种加密方式在某些场景下非常有用,比如需要对数据库中的数据进行排序和搜索,但是又需要保护数据的隐私。
常见的保序加密有两种:
1. 基于可逆加密算法的保序加密(如AES、DES等),它们使用的是块加密算法,在加密之后会得到固定长度的密文。
2. 基于非可逆加密算法的保序加密(如SHA-1、SHA-2等),它们使用的是哈希算法,加密之后得到的密文长度是固定的,但是由于哈希算法是不可逆的,所以无法还原出原始明文。
下面是基于可逆加密算法的保序加密的实现示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/aes.h>
// 加密函数
void encrypt(char *plaintext, char *key, char *ciphertext){
AES_KEY aes_key;
AES_set_encrypt_key(key, 128, &aes_key);
AES_encrypt(plaintext, ciphertext, &aes_key);
}
// 解密函数
void decrypt(char *ciphertext, char *key, char *plaintext){
AES_KEY aes_key;
AES_set_decrypt_key(key, 128, &aes_key);
AES_decrypt(ciphertext, plaintext, &aes_key);
}
// OPE 加密函数
void ope_encrypt(unsigned long long plaintext, char *key, unsigned long long *ciphertext){
// 将明文转换为字符串
char plaintext_str[100];
sprintf(plaintext_str, "%llu", plaintext);
// 加密字符串
char ciphertext_str[100];
encrypt(plaintext_str, key, ciphertext_str);
// 将密文转换为数字
*ciphertext = atoll(ciphertext_str);
}
// OPE 解密函数
void ope_decrypt(unsigned long long ciphertext, char *key, unsigned long long *plaintext){
// 将密文转换为字符串
char ciphertext_str[100];
sprintf(ciphertext_str, "%llu", ciphertext);
// 解密字符串
char plaintext_str[100];
decrypt(ciphertext_str, key, plaintext_str);
// 将明文转换为数字
*plaintext = atoll(plaintext_str);
}
int main(){
// 定义明文和密钥
unsigned long long plaintext = 123456789;
char *key = "0123456789abcdef";
// 加密明文
unsigned long long ciphertext;
ope_encrypt(plaintext, key, &ciphertext);
printf("明文:%llu,密文:%llu\n", plaintext, ciphertext);
// 解密密文
unsigned long long plaintext2;
ope_decrypt(ciphertext, key, &plaintext2);
printf("解密后的明文:%llu\n", plaintext2);
return 0;
}
```
这里使用了 OpenSSL 库中的 AES 加密算法实现 OPE 加密。需要注意的是,由于 OPE 加密只保证明文的大小关系不变,而不是保证明文的绝对值不变,所以加密之后的密文可能会很大,需要使用 unsigned long long 类型存储。
阅读全文
相关推荐
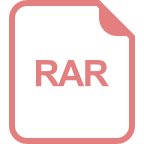
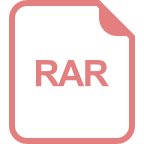
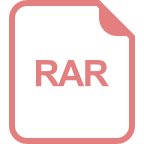
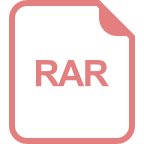
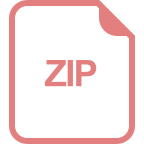
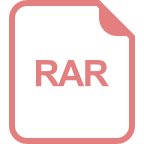
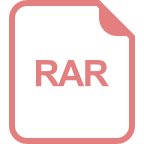
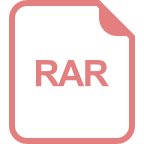
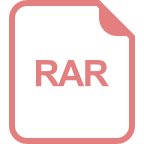
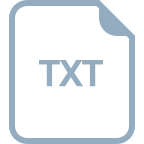
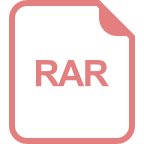
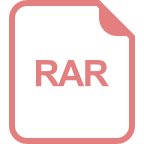
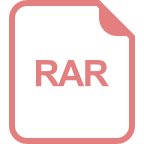
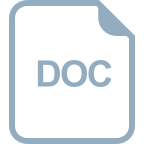

