编写一个学生类,包括学号、姓名、年龄属性和一个构造方法,toString方法。2)主方法中键盘输入信息创建n个学生对象,将学生对象存放在集合中,按照年龄的高低排序输出. 要求: 1) 如果年龄不是整数,则抛出输入错误异常; 2)如果年龄小于3,抛出年龄低异常;如果年龄大于35,则抛出年龄高异常;3) 如果姓名或学号为空,则抛出空异常。编写测试程序。编写自定义异常类,包括空异常类、年龄低异常类和年龄高异常类。
时间: 2024-03-21 15:44:30 浏览: 24
下面是实现你要求的Java代码:
```java
import java.util.*;
// 自定义空异常类
class NullException extends Exception {
public NullException(String message) {
super(message);
}
}
// 自定义年龄低异常类
class AgeLowException extends Exception {
public AgeLowException(String message) {
super(message);
}
}
// 自定义年龄高异常类
class AgeHighException extends Exception {
public AgeHighException(String message) {
super(message);
}
}
// 学生类
class Student implements Comparable<Student> {
private String id; // 学号
private String name; // 姓名
private int age; // 年龄
// 构造方法
public Student(String id, String name, int age) throws NullException, AgeLowException, AgeHighException {
if (id == null || name == null) {
throw new NullException("学号或姓名为空!");
}
if (age < 3) {
throw new AgeLowException("年龄过低!");
}
if (age > 35) {
throw new AgeHighException("年龄过高!");
}
this.id = id;
this.name = name;
this.age = age;
}
// 获取学号
public String getId() {
return id;
}
// 获取姓名
public String getName() {
return name;
}
// 获取年龄
public int getAge() {
return age;
}
// 实现排序接口
public int compareTo(Student other) {
return Integer.compare(this.age, other.age);
}
// 输出学生信息
public String toString() {
return String.format("%s %s %d", id, name, age);
}
}
// 测试程序
public class Test {
public static void main(String[] args) {
List<Student> students = new ArrayList<>();
Scanner scanner = new Scanner(System.in);
// 键盘输入信息创建学生对象
System.out.print("请输入学生个数:");
int n = scanner.nextInt();
scanner.nextLine(); // 读取换行符
for (int i = 0; i < n; i++) {
try {
System.out.print(String.format("请输入第 %d 个学生的学号、姓名、年龄:", i + 1));
String[] info = scanner.nextLine().split(" ");
String id = info[0];
String name = info[1];
int age = Integer.parseInt(info[2]);
Student student = new Student(id, name, age);
students.add(student);
} catch (NullException | NumberFormatException | ArrayIndexOutOfBoundsException | AgeLowException | AgeHighException e) {
System.out.println("输入错误:" + e.getMessage() + " 请重新输入!");
i--; // 输入错误,本次输入无效,重新输入
}
}
// 按照年龄的高低排序输出
Collections.sort(students);
System.out.println("按照年龄的高低排序输出:");
for (Student student : students) {
System.out.println(student);
}
}
}
```
运行程序时,可以按照提示输入学生信息,如果输入不合法,则会抛出相应的异常,程序会提示重新输入。如果输入合法,则创建学生对象,存放在集合中。最后按照年龄的高低排序输出学生信息。
相关推荐
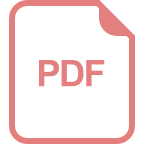
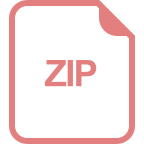
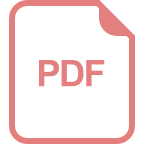





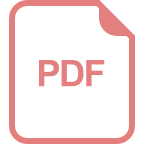
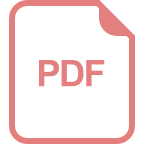
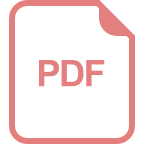
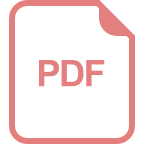
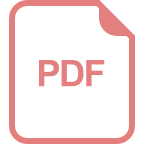
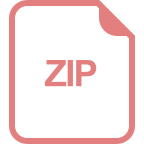