2. 编写一个学生类,包括学号、姓名、年龄属性和一个构造方法,toString方法。2)主方法种键盘输入信息创建n个学生对象,将学生对象存放在集合中,按照年龄的高低排序输出. 要求: 1) 如果年龄不是整数,则抛出输入错误异常; 2)如果年龄小于3,抛出年龄低异常;如果年龄大于35,则抛出年龄高异常;3) 如果姓名或学号为空,则抛出空异常。编写测试程序。编写自定义异常类,包括空异常类、年龄低异常类和年龄高异常类。
时间: 2024-02-19 21:02:42 浏览: 27
好的,我来回答你的问题。
下面是一个简单的Java代码,实现了你提出的需求,包括学生类、自定义异常类和测试程序。
```java
import java.util.*;
class Student {
private String id;
private String name;
private int age;
public Student(String id, String name, int age) throws AgeException, EmptyException {
if (id == null || id.equals("") || name == null || name.equals("")) {
throw new EmptyException("学号或姓名为空");
}
if (age < 3) {
throw new AgeLowException("年龄太低");
}
if (age > 35) {
throw new AgeHighException("年龄太高");
}
if (age != (int)age) {
throw new AgeException("年龄不是整数");
}
this.id = id;
this.name = name;
this.age = age;
}
public String getId() {
return id;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public String toString() {
return "学号:" + id + ",姓名:" + name + ",年龄:" + age;
}
}
class AgeException extends Exception {
public AgeException(String message) {
super(message);
}
}
class AgeLowException extends Exception {
public AgeLowException(String message) {
super(message);
}
}
class AgeHighException extends Exception {
public AgeHighException(String message) {
super(message);
}
}
class EmptyException extends Exception {
public EmptyException(String message) {
super(message);
}
}
public class Test {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.print("请输入学生数量:");
int n = sc.nextInt();
List<Student> list = new ArrayList<>();
for (int i = 0; i < n; i++) {
System.out.print("请输入学号:");
String id = sc.next();
System.out.print("请输入姓名:");
String name = sc.next();
System.out.print("请输入年龄:");
int age = 0;
try {
age = sc.nextInt();
Student stu = new Student(id, name, age);
list.add(stu);
} catch (AgeException e) {
System.out.println("年龄输入错误:" + e.getMessage());
} catch (AgeLowException e) {
System.out.println("年龄输入错误:" + e.getMessage());
} catch (AgeHighException e) {
System.out.println("年龄输入错误:" + e.getMessage());
} catch (EmptyException e) {
System.out.println("输入错误:" + e.getMessage());
} catch (InputMismatchException e) {
System.out.println("输入错误:年龄必须是整数");
sc.next();
}
}
Collections.sort(list, new Comparator<Student>() {
public int compare(Student s1, Student s2) {
return s2.getAge() - s1.getAge();
}
});
System.out.println("按年龄从高到低排序:");
for (Student stu : list) {
System.out.println(stu);
}
}
}
```
在这个程序中,我们定义了一个 `Student` 类来表示学生,它包括了学号、姓名、年龄属性和一个构造方法、`toString` 方法。我们还定义了四个自定义异常类,分别表示空异常、年龄低异常、年龄高异常和年龄输入错误异常。
在 `main` 方法中,我们使用了一个 `Scanner` 对象来读取键盘输入的信息,并创建了 `n` 个学生对象,将学生对象存放在集合中。在创建学生对象时,如果输入信息不符合要求,就会抛出相应的异常,并在控制台上输出错误信息。最后,我们使用 `Collections.sort` 方法按照年龄从高到低对学生集合进行排序,并输出每个学生对象的信息。
希望这个程序能够帮助你解决问题。如果你还有其他问题,可以继续问我。
相关推荐
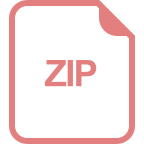
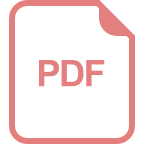
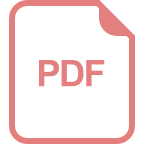




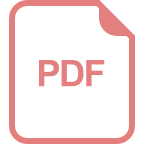
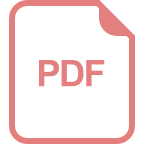
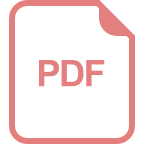
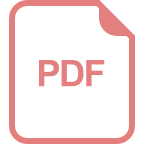
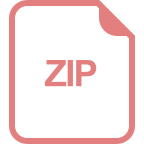
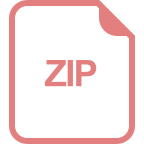