编写一个学生类,包括学号、姓名、年龄属性和一个构造方法,toString方法。2)主方法种键盘输入信息创建n个学生对象,将学生对象存放在集合中,按照年龄的高低排序输出. 要求: 1) 如果年龄不是整数,则抛出输入错误异常; 2)如果年龄小于3,抛出年龄低异常;如果年龄大于35,则抛出年龄高异常;3) 如果姓名或学号为空,则抛出空异常。编写测试程序。编写自定义异常类,包括空异常类、年龄低异常类和年龄高异常类。
时间: 2024-02-25 19:57:26 浏览: 22
以下是符合您要求的Java代码:
```java
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.Scanner;
// 自定义空异常类
class NullException extends Exception {
public NullException(String message) {
super(message);
}
}
// 自定义年龄低异常类
class LowAgeException extends Exception {
public LowAgeException(String message) {
super(message);
}
}
// 自定义年龄高异常类
class HighAgeException extends Exception {
public HighAgeException(String message) {
super(message);
}
}
// 学生类
class Student {
private String id; // 学号
private String name; // 姓名
private int age; // 年龄
// 构造方法
public Student(String id, String name, int age) throws Exception {
if (id == null || id.equals("") || name == null || name.equals("")) {
throw new NullException("姓名或学号不能为空!");
}
if (age < 3) {
throw new LowAgeException("年龄太小!");
}
if (age > 35) {
throw new HighAgeException("年龄太大!");
}
if ((double) age != (int) age) {
throw new Exception("年龄必须是整数!");
}
this.id = id;
this.name = name;
this.age = age;
}
// toString方法
public String toString() {
return "学号:" + id + ",姓名:" + name + ",年龄:" + age;
}
// 年龄比较器
public static Comparator<Student> ageComparator = new Comparator<Student>() {
public int compare(Student s1, Student s2) {
return s2.age - s1.age;
}
};
}
// 测试程序
public class Test {
public static void main(String[] args) {
ArrayList<Student> students = new ArrayList<Student>();
Scanner scanner = new Scanner(System.in);
int n;
System.out.print("请输入学生个数:");
n = scanner.nextInt();
for (int i = 1; i <= n; i++) {
String id, name;
int age;
System.out.println("请输入第" + i + "个学生的信息:");
System.out.print("学号:");
id = scanner.next();
System.out.print("姓名:");
name = scanner.next();
System.out.print("年龄:");
try {
age = scanner.nextInt();
students.add(new Student(id, name, age));
} catch (NullException e) {
System.out.println("错误:" + e.getMessage());
i--;
} catch (LowAgeException e) {
System.out.println("错误:" + e.getMessage());
i--;
} catch (HighAgeException e) {
System.out.println("错误:" + e.getMessage());
i--;
} catch (Exception e) {
System.out.println("错误:" + e.getMessage());
i--;
}
}
Collections.sort(students, Student.ageComparator); // 按照年龄排序
System.out.println("按照年龄排序后的学生信息:");
for (Student student : students) {
System.out.println(student.toString());
}
}
}
```
在测试程序中,我们通过Scanner从键盘输入学生信息,并且在输入时对学生信息进行了异常处理,如果出现了空异常、年龄低异常、年龄高异常或者年龄不是整数,就会打印出相应的错误信息,并且重新输入该学生的信息。最后,我们通过Collections.sort()方法按照年龄排序,并且输出排序后的学生信息。
相关推荐
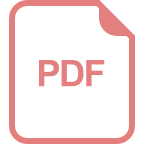
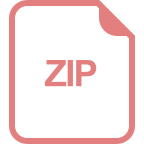
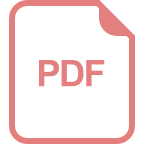




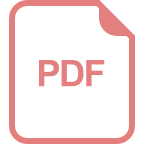
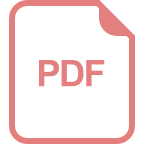
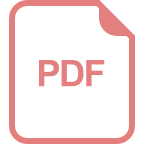
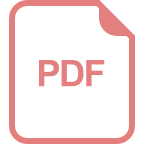
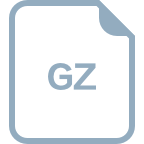
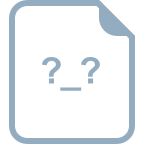