编写一个学生类,包括学号、姓名、年龄属性和一个构造方法,toString方法。2)主方法中键盘输入信息创建n个学生对象,将学生对象存放在集合中,按照年龄的高低排序输出. 要求: 1) 如果年龄不是整数,则抛出输入错误异常; 2)如果年龄小于3,抛出年龄低异常;如果年龄大于35,则抛出年龄高异常;3) 如果姓名或学号为空,则抛出空异常。编写测试程序。编写自定义异常类,包括空异常类、年龄低异常类和年龄高异常类。 给出解题思路
时间: 2024-03-22 17:37:08 浏览: 17
这道题目需要我们编写一个学生类,包括学号、姓名、年龄属性和一个构造方法,toString方法,并且需要自定义三种异常类:空异常类、年龄低异常类和年龄高异常类。然后在主方法中,键盘输入信息创建n个学生对象,将学生对象存放在集合中,按照年龄的高低排序输出。
下面是解题思路:
1. 编写学生类,包括学号、姓名、年龄属性和一个构造方法,toString方法。
```Java
public class Student {
private String id;
private String name;
private int age;
public Student(String id, String name, int age) throws InputErrorException {
if (id == null || name == null) {
throw new InputErrorException("学号或姓名为空");
}
if (age < 3) {
throw new AgeLowException("年龄太低");
}
if (age > 35) {
throw new AgeHighException("年龄太高");
}
// 判断年龄是否为整数
if (age != Math.floor(age)) {
throw new InputErrorException("年龄不是整数");
}
this.id = id;
this.name = name;
this.age = age;
}
public String getId() {
return id;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
@Override
public String toString() {
return "学生信息:" + "学号:" + id + ", 姓名:" + name + ", 年龄:" + age;
}
}
```
2. 编写自定义异常类,包括空异常类、年龄低异常类和年龄高异常类。
```Java
//空异常类
public class InputErrorException extends Exception {
public InputErrorException(String message) {
super(message);
}
}
//年龄低异常类
public class AgeLowException extends InputErrorException {
public AgeLowException(String message) {
super(message);
}
}
//年龄高异常类
public class AgeHighException extends InputErrorException {
public AgeHighException(String message) {
super(message);
}
}
```
3. 在主方法中,键盘输入信息创建n个学生对象,将学生对象存放在集合中,按照年龄的高低排序输出。
```Java
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int n = scanner.nextInt();
List<Student> list = new ArrayList<>();
for (int i = 0; i < n; i++) {
try {
String id = scanner.next();
String name = scanner.next();
int age = scanner.nextInt();
Student student = new Student(id, name, age);
list.add(student);
} catch (InputErrorException e) {
System.out.println(e.getMessage());
}
}
Collections.sort(list, (o1, o2) -> o2.getAge() - o1.getAge());
for (Student student : list) {
System.out.println(student.toString());
}
}
}
```
这样,我们就完成了题目要求。在输入时,如果学号或姓名为空,年龄太低或太高或年龄不是整数,将抛出相应的异常,并在主方法中进行捕捉处理,最后按照年龄的高低排序输出学生信息。
相关推荐
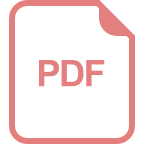
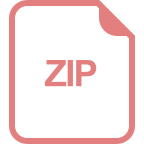
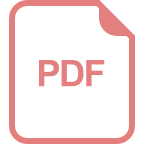




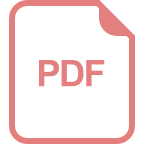
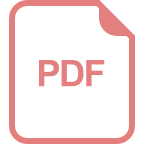
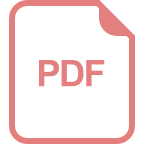
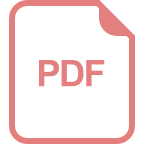
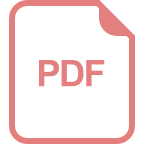
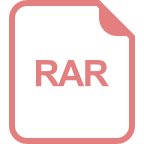
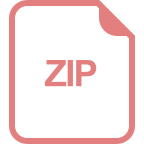