怎么根据用户输入的内容与类里所存的所有对象的属性进行对比,如果输入内容与类里的所有对象的属性均不同,则返回false,反之返回treu
时间: 2024-03-05 21:53:20 浏览: 23
你可以在 `get` 方法中使用一个循环遍历 `re` 数组,对于数组中的每个 `Reaction` 实例,以及用户输入的化学反应实例 `Test`,都比较它们的各个属性是否相等。如果 `Test` 与数组中的至少一个实例匹配,就可以返回 true;否则,当遍历完整个数组后,返回 false。
具体实现可以参考下面的代码:
```java
public static boolean get() {
String str = Frame.text.getText();
String str1 = Frame.text1.getText();
String str2 = Frame.saveValue;
String str3 = Frame.saveValue1;
System.out.println(str + str1 + str2 + str3);
Reaction[] test = new Reaction[1];
Reaction Test = new Reaction(str, str1, str2, str3);
test[0] = Test;
System.out.println(re[0]);
System.out.println(test[0]);
for (int i = 0; i < re.length; i++) {
Reaction target = re[i];
if (target.equals(Test)) { // 使用 Reaction 类中的 equals 方法比较两个实例是否相等
System.out.println("Matched: " + target);
return true;
}
}
System.out.println("No match found.");
return false;
}
```
在上面的代码中,我们使用了 `Reaction` 类中的 `equals` 方法来比较两个实例是否相等。你需要在 `Reaction` 类中实现这个方法,比较该类的各个属性是否都相等。例如:
```java
@Override
public boolean equals(Object obj) {
if (obj instanceof Reaction) {
Reaction other = (Reaction) obj;
if (Objects.equals(this.reactant1, other.reactant1)
&& Objects.equals(this.reactant2, other.reactant2)
&& Objects.equals(this.condition, other.condition)
&& Objects.equals(this.product, other.product)) {
return true;
}
}
return false;
}
```
在上面的代码中,我们首先检查传入的 `obj` 是否是 `Reaction` 类的实例。如果是,就将其转换为 `Reaction` 类型的变量 `other`,然后比较两个实例的各个属性是否都相等,如果都相等,则返回 true,否则返回 false。注意,我们使用了 `Objects.equals` 方法来比较字符串类型的属性是否相等,这样可以避免在某个属性为 null 时出现空指针异常。
相关推荐
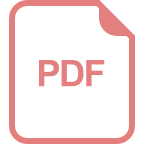
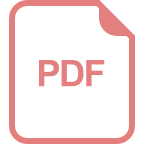
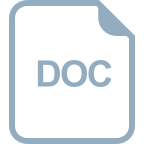














