c#dll嵌入dll并调用
时间: 2023-08-02 11:05:04 浏览: 65
在C#中嵌入DLL并调用其中的函数,可以使用DllImport属性和LoadLibrary函数。
以下是实现的步骤:
1. 创建一个C#项目, 添加一个类,并引入System.Runtime.InteropServices命名空间。
2. 使用DllImport属性声明要调用的DLL和其中的函数。例如:
```csharp
[DllImport("user32.dll")]
public static extern int MessageBox(IntPtr hWnd, string text, string caption, int options);
```
3. 在代码中使用该函数。例如:
```csharp
MessageBox(IntPtr.Zero, "Hello World!", "Greeting", 0);
```
4. 如果要嵌入DLL,可以使用LoadLibrary函数加载DLL,并获取其中的函数地址。例如:
```csharp
IntPtr handle = LoadLibrary("mydll.dll");
IntPtr funcPtr = GetProcAddress(handle, "myfunc");
```
5. 然后可以使用Marshal.GetDelegateForFunctionPointer方法将函数地址转换为委托类型,并使用该委托调用函数。
完整代码示例:
```csharp
using System;
using System.Runtime.InteropServices;
class Program
{
[DllImport("user32.dll")]
public static extern int MessageBox(IntPtr hWnd, string text, string caption, int options);
[DllImport("mydll.dll")]
public static extern int myfunc(int arg);
[DllImport("kernel32.dll")]
public static extern IntPtr LoadLibrary(string path);
[DllImport("kernel32.dll")]
public static extern IntPtr GetProcAddress(IntPtr handle, string symbol);
static void Main()
{
MessageBox(IntPtr.Zero, "Hello World!", "Greeting", 0);
IntPtr handle = LoadLibrary("mydll.dll");
IntPtr funcPtr = GetProcAddress(handle, "myfunc");
var myFunc = Marshal.GetDelegateForFunctionPointer<myfuncDelegate>(funcPtr);
int result = myFunc(42);
Console.WriteLine($"Result: {result}");
}
[UnmanagedFunctionPointer(CallingConvention.Cdecl)]
delegate int myfuncDelegate(int arg);
}
```
相关推荐
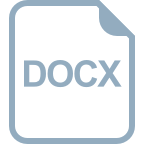
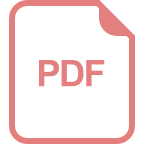














