python 正则匹配url中的域名
时间: 2024-05-17 15:17:51 浏览: 14
可以使用Python中的re模块来进行正则匹配。下面是一个例子:
```python
import re
url = "https://www.baidu.com/s?wd=python"
# 使用正则表达式匹配域名
pattern = r"(https?://)?(www\.)?([a-zA-Z0-9-]+)\.[a-zA-Z]+(/[a-zA-Z0-9-]*)*"
match = re.search(pattern, url)
if match:
domain = match.group(3)
print(domain)
else:
print("No domain found in the URL.")
```
在上面的代码中,我们使用正则表达式`"(https?://)?(www\.)?([a-zA-Z0-9-]+)\.[a-zA-Z]+(/[a-zA-Z0-9-]*)*"`来匹配URL中的域名。其中,`([a-zA-Z0-9-]+)\.[a-zA-Z]+`匹配域名部分,`([a-zA-Z0-9-]+)`表示域名中可以包含字母、数字和横线,`\.`表示匹配点号,`[a-zA-Z]+`表示匹配顶级域名,例如`.com`、`.cn`等。`(/[a-zA-Z0-9-]*)*`表示匹配URL中的路径部分,用于处理类似于`https://www.baidu.com/s`这样的URL。如果匹配成功,则输出域名部分。
相关问题
python正则匹配域名
可以使用Python的re模块来匹配域名。以下是一个简单的示例代码:
```python
import re
url = "https://www.google.com"
pattern = r"https?://([\w\-\.]+)"
match = re.search(pattern, url)
if match:
domain = match.group(1)
print(domain)
else:
print("No match")
```
输出结果为:
```
www.google.com
```
在上面的代码中,我们使用正则表达式模式`https?://([\w\-\.]+)`来匹配以http或https开头的URL,然后提取域名部分。其中:
- `https?`表示匹配http或https协议;
- `([\w\-\.]+)`表示匹配包含字母、数字、下划线、连字符和点号的字符串,其中点号需要用反斜杠转义,表示匹配域名中的点号。
如果匹配成功,则使用`group(1)`方法提取第一个括号内的内容,即域名部分。
python正则匹配字符串
好的,对于Python正则表达式匹配字符串,我可以为您提供一些解释和示例。正则表达式是一种用于匹配字符串的工具,能够从字符串中找到所需的子串。在Python中,可以使用re模块来实现正则表达式匹配。
例如,假设我们想从一个URL中提取域名,可以使用以下代码:
import re
url = "https://www.google.com/search?q=python"
pattern = r"https?://([\w\.]+)/"
match = re.search(pattern, url)
if match:
print(match.group(1))
运行此代码,输出结果为“www.google.com”。
需要注意的是,正则表达式的语法比较复杂,在匹配字符串时需要仔细检查模式是否正确,否则可能无法匹配预期的字符串。
相关推荐
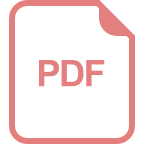
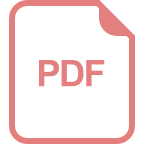
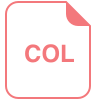
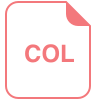
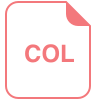
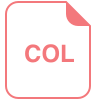
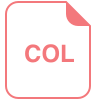







