解释clf = SVM(C=1, kernel=Kernel.linear()) clf.fit(train_set_x, train_set_y) y_pred = clf.predict(test_set_x) accuracy(y_pred, test_set_y)
时间: 2023-12-24 15:06:34 浏览: 180
这段代码使用支持向量机(SVM)算法对训练集数据进行训练,然后使用训练好的模型对测试集数据进行预测,并计算预测结果的准确率。
具体解释如下:
- `clf = SVM(C=1, kernel=Kernel.linear())`:创建一个支持向量机实例,使用线性核函数,正则化系数为1。
- `clf.fit(train_set_x, train_set_y)`:使用训练集数据进行拟合,训练出一个SVM模型。
- `y_pred = clf.predict(test_set_x)`:使用训练好的模型对测试集数据进行预测,得到预测结果。
- `accuracy(y_pred, test_set_y)`:计算预测结果的准确率,其中`y_pred`为预测结果,`test_set_y`为测试集数据的真实标签。
相关问题
优化代码import numpy as np from PIL import Image from sklearn import svm from sklearn.model_selection import train_test_split import os import matplotlib.pyplot as plt # 定义图像文件夹路径和类别 cat_path = "cats/" dog_path = "dogs/" cat_label = 0 dog_label = 1 # 定义图像预处理函数 def preprocess_image(file_path): # 读取图像并转换为灰度图像 img = Image.open(file_path).convert('L') # 调整图像尺寸 img = img.resize((100, 100)) # 将图像转换为 Numpy 数组 img_array = np.array(img) # 将二维数组展平为一维数组 img_array = img_array.reshape(-1) return img_array # 读取猫和狗的图像并转换成 Numpy 数组 X = [] y = [] for file_name in os.listdir(cat_path): file_path = os.path.join(cat_path, file_name) img_array = preprocess_image(file_path) X.append(img_array) y.append(cat_label) for file_name in os.listdir(dog_path): file_path = os.path.join(dog_path, file_name) img_array = preprocess_image(file_path) X.append(img_array) y.append(dog_label) X = np.array(X) y = np.array(y) # 将数据集划分为训练集和测试集 X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3) # 训练 SVM 分类器 clf = svm.SVC(kernel='linear') clf.fit(X_train, y_train) # 在测试集上进行预测 y_pred = clf.predict(X_test) # 计算测试集上的准确率 accuracy = np.mean(y_pred == y_test) print("Accuracy:", accuracy) # 显示测试集中的前 16 张图像和它们的预测结果 fig, axes = plt.subplots(nrows=4, ncols=4, figsize=(8, 8)) for i, ax in enumerate(axes.flat): # 显示图像 ax.imshow(X_test[i].reshape(100, 100), cmap='gray') # 显示预测结果和标签 if y_pred[i] == 0: ax.set_xlabel("Cat") else: ax.set_xlabel("Dog") ax.set_xticks([]) ax.set_yticks([]) plt.show()
这段代码已经很简洁了,不过还有一些可以优化的地方:
1. 在导入模块时,可以将不同模块分行导入,以提高代码可读性。
2. 在预处理函数中,可以将调整图像尺寸和转换为 Numpy 数组合并为一步,以减少代码行数。
3. 在循环读取图像并转换为 Numpy 数组时,可以使用列表推导式代替 for 循环,以提高代码效率。
4. 在计算准确率时,可以使用 sklearn.metrics 中的 accuracy_score 函数代替自己编写的代码。
下面是优化后的代码:
```python
import numpy as np
from PIL import Image
from sklearn import svm, metrics
from sklearn.model_selection import train_test_split
import os
import matplotlib.pyplot as plt
# 定义图像文件夹路径和类别
cat_path = "cats/"
dog_path = "dogs/"
cat_label = 0
dog_label = 1
# 定义图像预处理函数
def preprocess_image(file_path):
# 读取图像并转换为灰度图像,调整尺寸并转换为 Numpy 数组
img = np.array(Image.open(file_path).convert('L').resize((100, 100)))
# 将二维数组展平为一维数组
img_array = img.reshape(-1)
return img_array
# 读取猫和狗的图像并转换成 Numpy 数组
cat_files = [os.path.join(cat_path, f) for f in os.listdir(cat_path)]
dog_files = [os.path.join(dog_path, f) for f in os.listdir(dog_path)]
X = [preprocess_image(f) for f in cat_files + dog_files]
y = np.concatenate([np.full(len(cat_files), cat_label), np.full(len(dog_files), dog_label)])
# 将数据集划分为训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3)
# 训练 SVM 分类器
clf = svm.SVC(kernel='linear')
clf.fit(X_train, y_train)
# 在测试集上进行预测
y_pred = clf.predict(X_test)
# 计算测试集上的准确率
accuracy = metrics.accuracy_score(y_test, y_pred)
print("Accuracy:", accuracy)
# 显示测试集中的前 16 张图像和它们的预测结果
fig, axes = plt.subplots(nrows=4, ncols=4, figsize=(8, 8))
for i, ax in enumerate(axes.flat):
# 显示图像
ax.imshow(X_test[i].reshape(100, 100), cmap='gray')
# 显示预测结果和标签
if y_pred[i] == 0:
ax.set_xlabel("Cat")
else:
ax.set_xlabel("Dog")
ax.set_xticks([])
ax.set_yticks([])
plt.show()
```
import numpy as np import matplotlib.pyplot as plt from sklearn import svm from sklearn.datasets import make_blobs from sklearn import model_selection from sklearn.metrics import f1_score def show_svm(a, b, bt): plt.figure(bt) plt.title('SVM with ' + bt) # 建立图像坐标 axis = plt.gca() plt.scatter(a[:, 0], a[:, 1], c=b, s=30) xlim = [a[:, 0].min(), a[:, 0].max()] ylim = [a[:, 1].min(), a[:, 1].max()] # 生成两个等差数列 xx = np.linspace(xlim[0], xlim[1], 50) yy = np.linspace(ylim[0], ylim[1], 50) X, Y = np.meshgrid(xx, yy) xy = np.vstack([X.ravel(), Y.ravel()]).T Z = clf.decision_function(xy).reshape(X.shape) # 画出分界线 axis.contour(X, Y, Z, colors='k', levels=[-1, 0, 1], alpha=0.5, linestyles=['--', '-', '--']) axis.scatter(clf.support_vectors_[:, 0], clf.support_vectors_[:, 1], s=200, linewidths=1, facecolors='none') if __name__ == '__main__': # data = np.loadtxt('separable_data.txt', delimiter=',') # data = np.loadtxt('non_separable_data.txt', delimiter=',') # data = np.loadtxt('banknote.txt', delimiter=',') data = np.loadtxt('ionosphere.txt', delimiter=',') # data = np.loadtxt('wdbc.txt', delimiter=',') X = data[:, 0:-1] y = data[:, -1] """标签中有一类标签为1""" y = y + 1 ymin = min(y) if not (1 in set(y)): ll = max(list(set(y))) + 1 for i in range(len(y)): if y[i] == ymin: y[i] = 1 # 建立一个线性核(多项式核)的SVM clf = svm.SVC(kernel='linear') clf.fit(X, y) """显示所有数据用于训练后的可视化结果""" show_svm(X, y, 'all dataset') """divide the data into two sections: training and test datasets""" X_train, X_test, y_train, y_test = model_selection.train_test_split(X, y, test_size=0.1, random_state=42) """training""" clf = svm.SVC(kernel='linear')#线性内核 # clf = svm.SVC(kernel='poly')# 多项式内核 # clf = svm.SVC(kernel='sigmoid')# Sigmoid内核 clf.fit(X_train, y_train) # show_svm(X_train, y_train, 'training dataset') """predict""" pred = clf.predict(X_test) pred = np.array(pred) y_test = np.array(y_test) print(f'SVM 的预测结果 f1-score:{f1_score(y_test, pred)}') # plt.show()结果与分析
这段代码实现了一个支持向量机(SVM)分类器,并对数据进行了可视化展示。具体来说,它包括以下几个主要步骤:
1. 导入需要使用的库,如 numpy、matplotlib、sklearn 等。
2. 定义一个名为 show_svm 的函数,用于对数据进行可视化展示。函数有三个参数:a 表示数据的特征值,b 表示数据的标签,bt 表示图像的标题。
3. 在主函数中,首先读取数据,然后将标签中的一类标签设为 1,并划分数据集为训练集和测试集。
4. 建立一个线性核的 SVM 分类器,并用训练集进行训练。
5. 对训练后的模型进行可视化展示,包括所有数据集和训练数据集的分界线。
6. 对测试集进行预测,并计算预测结果的 f1-score。
7. 最后展示结果。
总的来说,这段代码的功能是对数据进行 SVM 分类,并对分类结果进行可视化和评估。其中,SVM 模型的核函数可以通过修改代码中的 kernel 参数进行更换。
阅读全文
相关推荐
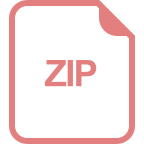
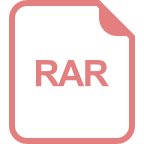
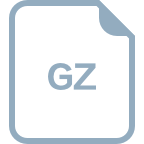













