python手写数字识别代码
时间: 2023-09-15 10:23:15 浏览: 145
下面是一个简单的 Python 代码,用于手写数字识别。它使用了一个简单的神经网络模型,该模型由一个输入层、一个隐藏层和一个输出层组成。
```python
import numpy as np
import matplotlib.pyplot as plt
import tensorflow as tf
from tensorflow.examples.tutorials.mnist import input_data
# 加载 MNIST 数据集
mnist = input_data.read_data_sets("MNIST_data/", one_hot=True)
# 定义神经网络的模型
input_size = 784
hidden_size = 100
output_size = 10
x = tf.placeholder(tf.float32, [None, input_size])
y = tf.placeholder(tf.float32, [None, output_size])
W1 = tf.Variable(tf.truncated_normal([input_size, hidden_size], stddev=0.1))
b1 = tf.Variable(tf.zeros([hidden_size]))
W2 = tf.Variable(tf.truncated_normal([hidden_size, output_size], stddev=0.1))
b2 = tf.Variable(tf.zeros([output_size]))
hidden_layer = tf.nn.relu(tf.matmul(x, W1) + b1)
output_layer = tf.matmul(hidden_layer, W2) + b2
# 定义损失函数和优化器
loss = tf.reduce_mean(tf.nn.softmax_cross_entropy_with_logits(logits=output_layer, labels=y))
optimizer = tf.train.AdamOptimizer().minimize(loss)
# 训练模型
batch_size = 100
num_epochs = 10
num_batches = mnist.train.num_examples // batch_size
with tf.Session() as sess:
sess.run(tf.global_variables_initializer())
for epoch in range(num_epochs):
for batch in range(num_batches):
batch_x, batch_y = mnist.train.next_batch(batch_size)
sess.run(optimizer, feed_dict={x: batch_x, y: batch_y})
# 每个 epoch 结束后,计算模型在验证集上的准确率
valid_accuracy = np.mean(np.argmax(mnist.validation.labels, axis=1) == sess.run(tf.argmax(output_layer, axis=1), feed_dict={x: mnist.validation.images}))
print("Epoch {} - Validation Accuracy: {:.4f}".format(epoch + 1, valid_accuracy))
# 在测试集上评估模型的准确率
test_accuracy = np.mean(np.argmax(mnist.test.labels, axis=1) == sess.run(tf.argmax(output_layer, axis=1), feed_dict={x: mnist.test.images}))
print("Test Accuracy: {:.4f}".format(test_accuracy))
# 随机选择一个测试样本进行预测,并可视化预测结果
idx = np.random.randint(mnist.test.images.shape[0])
img = mnist.test.images[idx].reshape([28, 28])
plt.imshow(img, cmap="gray")
plt.show()
pred = sess.run(tf.argmax(output_layer, axis=1), feed_dict={x: [mnist.test.images[idx]]})
print("Model Prediction: {}".format(pred[0]))
```
这个代码使用了 TensorFlow 框架,通过加载 MNIST 数据集进行训练和测试。在训练过程中,模型在每个 epoch 结束后在验证集上进行评估,以便监控模型的性能。在测试阶段,模型在测试集上进行评估,以获得最终的准确率。最后,我们从测试集中随机选择一个样本,并使用训练好的模型对其进行预测。
阅读全文
相关推荐
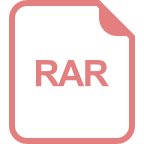
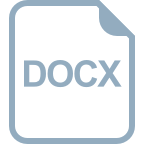



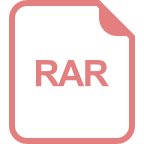
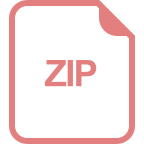
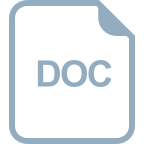
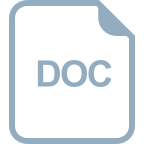
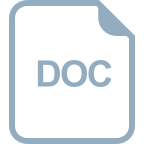






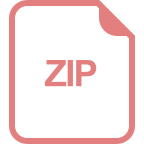
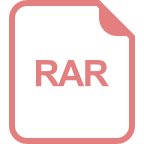