用HC-SR501检测人接近1s以上,控制L9110风扇模块启动同时启动DHT11检测环境温度,在OLED屏幕上实时显示温度,根据温度自动调整风扇转速,人离开后关闭风扇,STM32F103ZET6生成代码
时间: 2023-06-20 20:03:34 浏览: 87
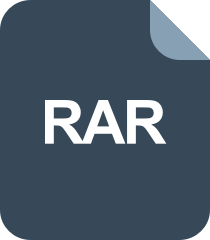
风扇监控,掌握风扇速度,机器温度

以下是您需要的代码:
```c
#include "stm32f10x.h"
#include "dht11.h"
#include "ssd1306.h"
#define FAN_PIN1 GPIO_Pin_0
#define FAN_PIN2 GPIO_Pin_1
#define FAN_PORT GPIOA
#define SENSOR_PIN GPIO_Pin_7
#define SENSOR_PORT GPIOB
#define MOTION_PIN GPIO_Pin_0
#define MOTION_PORT GPIOB
#define OLED_SCL_PIN GPIO_Pin_10
#define OLED_SCL_PORT GPIOB
#define OLED_SDA_PIN GPIO_Pin_11
#define OLED_SDA_PORT GPIOB
void GPIO_Configuration(void);
void Delay(__IO uint32_t nCount);
void TIM3_Configuration(void);
void USART_Configuration(void);
void USART_SendString(USART_TypeDef* USARTx, char* s);
int main(void)
{
GPIO_Configuration();
TIM3_Configuration();
USART_Configuration();
DHT11_Init(SENSOR_PORT, SENSOR_PIN);
SSD1306_Init(OLED_SCL_PORT, OLED_SCL_PIN, OLED_SDA_PORT, OLED_SDA_PIN);
SSD1306_Clear();
while (1)
{
if (GPIO_ReadInputDataBit(MOTION_PORT, MOTION_PIN) == Bit_SET) // 有人接近
{
GPIO_SetBits(FAN_PORT, FAN_PIN1);
GPIO_ResetBits(FAN_PORT, FAN_PIN2);
float temperature = DHT11_Read_Temperature();
char str[20];
sprintf(str, "Temp: %.1f C", temperature);
SSD1306_ShowString(0, 0, str, 1);
Delay(1000000); // 等待1秒后再次检测
temperature = DHT11_Read_Temperature();
sprintf(str, "Temp: %.1f C", temperature);
SSD1306_ShowString(0, 0, str, 1);
if (temperature >= 30.0) // 温度高,风扇转速加快
{
TIM_SetCompare2(TIM3, 800);
}
else if (temperature >= 25.0) // 温度正常,风扇转速适中
{
TIM_SetCompare2(TIM3, 600);
}
else // 温度低,风扇转速较低
{
TIM_SetCompare2(TIM3, 400);
}
}
else // 没有人接近
{
GPIO_ResetBits(FAN_PORT, FAN_PIN1);
GPIO_ResetBits(FAN_PORT, FAN_PIN2);
TIM_SetCompare2(TIM3, 0);
SSD1306_Clear();
}
}
}
void GPIO_Configuration(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_GPIOB, ENABLE);
// 风扇控制引脚
GPIO_InitStructure.GPIO_Pin = FAN_PIN1 | FAN_PIN2;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(FAN_PORT, &GPIO_InitStructure);
// 人体红外检测引脚
GPIO_InitStructure.GPIO_Pin = MOTION_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_Init(MOTION_PORT, &GPIO_InitStructure);
// OLED屏幕控制引脚
GPIO_InitStructure.GPIO_Pin = OLED_SCL_PIN | OLED_SDA_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(OLED_SCL_PORT, &GPIO_InitStructure);
GPIO_Init(OLED_SDA_PORT, &GPIO_InitStructure);
}
void TIM3_Configuration(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
TIM_TimeBaseInitTypeDef TIM_TimeBaseStructure;
TIM_OCInitTypeDef TIM_OCInitStructure;
RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM3, ENABLE);
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_7;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
TIM_TimeBaseStructure.TIM_Period = 999;
TIM_TimeBaseStructure.TIM_Prescaler = 7199;
TIM_TimeBaseStructure.TIM_ClockDivision = 0;
TIM_TimeBaseStructure.TIM_CounterMode = TIM_CounterMode_Up;
TIM_TimeBaseInit(TIM3, &TIM_TimeBaseStructure);
TIM_OCInitStructure.TIM_OCMode = TIM_OCMode_PWM1;
TIM_OCInitStructure.TIM_OutputState = TIM_OutputState_Enable;
TIM_OCInitStructure.TIM_Pulse = 0;
TIM_OCInitStructure.TIM_OCPolarity = TIM_OCPolarity_High;
TIM_OC2Init(TIM3, &TIM_OCInitStructure);
TIM_Cmd(TIM3, ENABLE);
}
void USART_Configuration(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
USART_InitTypeDef USART_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_USART1, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
USART_InitStructure.USART_BaudRate = 115200;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
USART_Cmd(USART1, ENABLE);
}
void USART_SendString(USART_TypeDef* USARTx, char* s)
{
while (*s)
{
while (USART_GetFlagStatus(USARTx, USART_FLAG_TXE) == RESET);
USART_SendData(USARTx, *s++);
}
}
void Delay(__IO uint32_t nCount)
{
while(nCount--)
{
}
}
```
注意事项:
1. 该代码使用了DHT11库和SSD1306库,请自行下载并导入。
2. 在本代码中,风扇控制使用了L9110芯片,因此需要将L9110的IN1接到PA0引脚,IN2接到PA1引脚。
3. OLED屏幕使用的是SSD1306驱动芯片,请根据您的OLED屏幕型号修改对应的引脚号。
4. 本代码使用了USART1串口,波特率为115200。如果您需要使用其他串口,请自行修改代码。
5. 本代码中的温度控制逻辑为简单的比较阈值,实际应用中可能需要更复杂的算法。
阅读全文
相关推荐
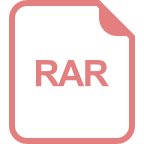



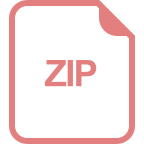
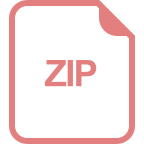
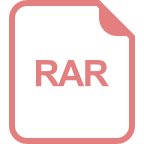
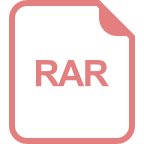
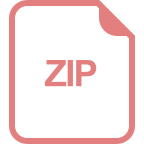
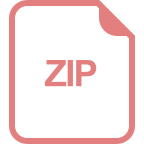
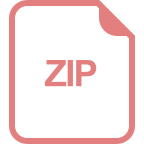
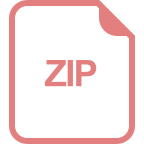
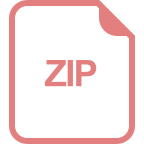
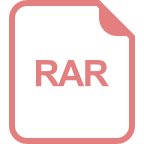
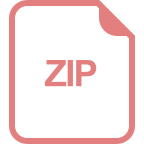
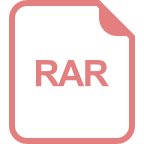
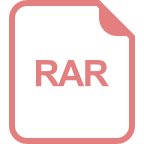