题目要求编写 C 语言程序,输入半径和高度,计算并输出圆周长、圆面积、球体积、圆柱体面积。
时间: 2024-12-06 18:23:03 浏览: 27
在C语言中,为了满足题目要求,你可以创建一个结构体来存储各种几何形状的相关信息,然后分别编写函数来计算每个形状的属性。以下是基本的步骤:
1. 定义一个包含圆周长(Circumference)、面积(Area)和体积(Volume)等字段的结构体,例如`Shape`:
```c
typedef struct {
double radius;
double height; // 只对圆柱体有效
} Shape;
```
2. 创建一个函数`calculate_circle`计算圆相关的值,包括圆周长和面积:
```c
double calculate_circle(Shape shape) {
const double PI = 3.14159;
return 2 * PI * shape.radius;
}
double calculate_circle_area(Shape shape) {
return PI * pow(shape.radius, 2);
}
```
3. 对于圆柱体,额外增加一个计算体积的函数`calculate_cylinder_volume`,并且需要考虑高度:
```c
double calculate_cylinder_volume(Shape cylinder) {
return M_PI * pow(cylinder.radius, 2) * cylinder.height;
}
```
4. 创建一个主函数,接收用户输入,创建`Shape`实例,并根据选择显示结果:
```c
void main() {
Shape circle;
printf("Enter the radius of the circle: ");
scanf("%lf", &circle.radius);
Shape cylinder;
printf("For a cylinder (enter radius and height): ");
scanf("%lf %lf", &cylinder.radius, &cylinder.height);
printf("Circle circumference: %.2f\n", calculate_circle(circle));
printf("Circle area: %.2f\n", calculate_circle_area(circle));
if (cylinder.height > 0) {
printf("Cylinder volume: %.2f\n", calculate_cylinder_volume(cylinder));
} else {
printf("Cylinder volume not applicable without height.\n");
}
}
```
阅读全文
相关推荐
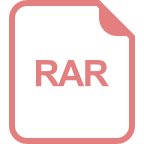
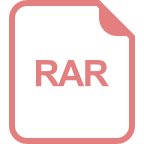
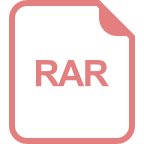















