怎么为结构体指针申请一块动态地址
时间: 2024-10-25 07:12:04 浏览: 17
在C语言中,为结构体指针申请动态内存通常涉及到`malloc()`或`calloc()`函数。以下是基本步骤:
1. 首先定义结构体:
```c
typedef struct {
int field1;
char field2[20];
} MyStruct;
```
2. 然后使用`malloc()`或`calloc()`为结构体分配内存:
```c
MyStruct* ptr = malloc(sizeof(MyStruct)); // 使用malloc
// 或者
MyStruct* ptr = calloc(1, sizeof(MyStruct)); // 使用calloc,同时分配并初始化为零
```
`malloc()`会返回一个指向新分配内存的`void*`指针,你需要强制转换为正确的类型。`calloc()`则会在分配内存的同时将其内容设置为0。
3. 检查分配是否成功:
```c
if (ptr == NULL) {
printf("Memory allocation failed.\n");
// 此处处理失败情况,比如释放已分配的内存
exit(1);
}
```
4. 初始化结构体:
```c
ptr->field1 = 123;
strcpy(ptr->field2, "example"); // 注意这里的strncpy如果字段2超过20个字节需要避免缓冲区溢出
```
5. 使用完后记得释放内存:
```c
free(ptr); // 释放动态分配的内存
```
相关问题
编写一个C语言程序执行内存申请系统调用,为可变分区管理算法设计空闲块单向链表,每个节点登记一块空闲块信息:块起始地址、块长度。请构造有3个节点(数据自定)的空闲块链表,最后输出这个链表的全部信息。提示:每个节点可以用一个结构体变量表示,比如: struct block{ int address; //块起始地址 int size; //块大小 struct block* next; //链指针 }oneBlock;
好的,我理解了你的问题。以下是实现内存申请系统调用的C语言程序,同时更新空闲块链表:
```c
#include <stdio.h>
#include <stdlib.h>
struct block {
int address; //块起始地址
int size; //块大小
struct block* next; //链指针
};
struct block* createBlock(int addr, int size) {
struct block* newBlock = (struct block*)malloc(sizeof(struct block));
newBlock->address = addr;
newBlock->size = size;
newBlock->next = NULL;
return newBlock;
}
void printBlockList(struct block* head) {
struct block* current = head;
while (current != NULL) {
printf("Block address: %d\tBlock size: %d\n", current->address, current->size);
current = current->next;
}
}
void allocateMemory(struct block* head, int size) {
struct block* previous = head;
struct block* current = head;
while (current != NULL) {
if (current->size >= size) {
if (current->size == size) {
previous->next = current->next;
} else {
current->address += size;
current->size -= size;
}
printf("Memory allocated at address %d with size %d\n", current->address - size, size);
return;
}
previous = current;
current = current->next;
}
printf("Memory allocation failed\n");
}
int main() {
struct block* head = createBlock(1000, 100);
head->next = createBlock(1200, 50);
head->next->next = createBlock(1300, 80);
printBlockList(head);
allocateMemory(head, 30);
allocateMemory(head, 60);
allocateMemory(head, 100);
printBlockList(head);
return 0;
}
```
这段程序定义了一个名为`block`的结构体,用于存储空闲块的信息。`createBlock`函数用于创建一个新的空闲块节点,并返回其地址。`printBlockList`函数用于遍历并输出完整的空闲块链表信息。
`allocateMemory`函数用于执行内存申请系统调用,同时更新空闲块链表。该函数接受空闲块链表头节点和所需内存大小作为参数。它会遍历整个链表,找到第一个足够大的空闲块,并将其划分为两部分(一部分被分配,另一部分仍然是空闲块),然后输出分配的内存块的地址和大小。如果没有足够大的空闲块,则输出内存申请失败的消息。
在`main`函数中,我们手动创建了一个包含3个节点的空闲块链表,并使用`printBlockList`函数输出了链表中的每个节点的信息。然后,我们连续执行了3次`allocateMemory`函数,以模拟3次内存申请操作,每次输出申请结果后再次使用`printBlockList`函数输出更新后的空闲块链表信息。你可以根据自己的需求修改这些节点和内存申请操作。
使用C语言代码实现以下需求 1、使用结构体和数组管理变量 2、动态申请一片内存,分为8个部分,每个部分的大小为800KB 3、在这8个部分中,每一部分可分为1024块,每一块需要存放一个int型数据
以下是使用C语言代码实现以上需求的示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义结构体
typedef struct {
int* data; // 指向数据的指针
int size; // 数据大小
} Block;
// 定义数组
typedef struct {
Block* blocks; // 指向块数组的指针
int count; // 块数量
} Array;
int main() {
// 动态申请内存
int blockCount = 1024;
int blockSize = 800 * 1024;
int totalSize = blockCount * blockSize;
int* memory = malloc(totalSize);
// 初始化数组
Array array;
array.count = 8;
array.blocks = malloc(sizeof(Block) * array.count);
// 将内存分为8块
for (int i = 0; i < array.count; i++) {
array.blocks[i].data = memory + i * blockSize;
array.blocks[i].size = blockSize / sizeof(int);
}
// 在每一块中存放数据
for (int i = 0; i < array.count; i++) {
for (int j = 0; j < array.blocks[i].size; j++) {
array.blocks[i].data[j] = i * array.blocks[i].size + j;
}
}
// 输出数据
for (int i = 0; i < array.count; i++) {
printf("Block %d:\n", i);
for (int j = 0; j < array.blocks[i].size; j++) {
printf("%d ", array.blocks[i].data[j]);
}
printf("\n");
}
// 释放内存
free(array.blocks);
free(memory);
return 0;
}
```
以上代码中,我们使用了两个结构体来管理变量。`Block`结构体表示内存中的一块,包括指向数据的指针和数据大小。`Array`结构体表示内存中的8块,包括指向块数组的指针和块数量。我们使用`malloc`函数动态申请了一片内存,并将其分为8块,每一块又分为1024个int型数据块。然后,我们在每一块中存放数据,并输出结果。最后,我们使用`free`函数释放了内存。
阅读全文
相关推荐
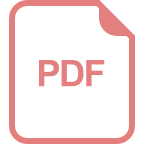
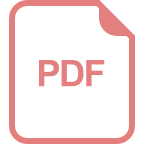
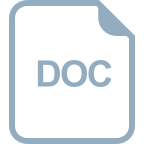
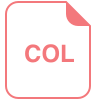
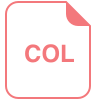
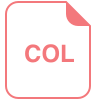
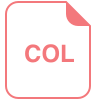
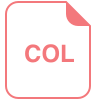
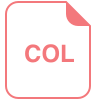
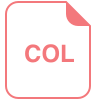
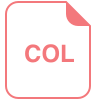





