从前往后查找子字符串
时间: 2024-10-12 13:09:53 浏览: 6
在计算机编程中,从前往后查找子字符串通常是指在一个主字符串(也称为父串或大串)中搜索指定的子串。这个过程可以使用多种方法实现,比如在Python中,你可以使用`str.find()`、`str.index()` 或者 `str.startswith()` 函数来进行查找。
例如,在Python中,如果你有一个主字符串`text = "Hello, World!"`,你想查找子字符串`"World"`,你可以这样做:
```python
main_str = "Hello, World!"
substring = "World"
# 使用find()函数
position = main_str.find(substring)
if position != -1:
print(f"'{substring}' found at index {position}")
else:
print("Substring not found")
# 使用startswith()
if main_str.startswith(substring):
print("'{}' starts with '{}'".format(main_str, substring))
```
这些函数会返回子串在主串中的起始位置索引,如果没有找到则返回特定值(如Python中的-1)。如果需要寻找所有出现的位置,可以结合循环多次调用查找方法。
相关问题
从前往后查找子字符串的c语言函数
在 C 语言中,如果你想要从前往后查找子字符串,可以使用标准库函数 `strstr()`。这个函数原型通常定义在 `<string.h>` 头文件中,它的作用是在给定的主字符串(也称为"haystack")中查找指定的子字符串("needle"),并返回第一次找到子字符串的位置指针。
`strstr(haystack, needle)` 的工作原理是:
1. 检查 `haystack` 是否以 `needle` 开始。如果是,它会返回 `haystack` 的地址。
2. 如果不是,它会在 `haystack` 的剩余部分继续搜索 `needle`。
3. 如果在整个字符串中都没有找到 `needle`,则返回 NULL 表示未找到。
这是一个基本的例子:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str[] = "Hello, World!";
char substr[] = "World";
char* result = strstr(str, substr);
if (result) {
printf("Substring found at position %ld\n", (long) result - (long) str); // 注意这里减去str的地址得到偏移量
} else {
printf("Substring not found.\n");
}
return 0;
}
```
python str find 从后往前找
Python中的字符串方法`find()`可以从前往后查找子字符串,如果要从后往前找,可以使用`rfind()`方法。`rfind()`的用法与`find()`相同,只不过是从字符串的末尾开始查找。例如:
```
s = "hello world"
print(s.rfind("l")) # 输出9,即最后一个字符"l"的索引
```
如果要查找所有匹配的子字符串,可以使用正则表达式或者`re`模块的`findall()`方法。例如:
```python
import re
s = "hello world"
matches = re.findall("l", s)
print(matches) # 输出['l', 'l']
```
这样可以找到所有的字符"l",而不仅仅是最后一个。
阅读全文
相关推荐
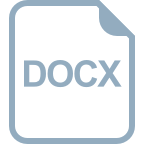
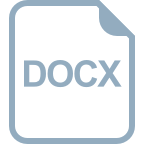
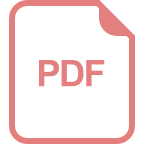
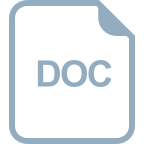
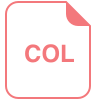
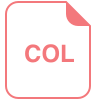
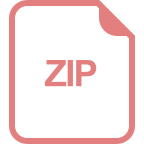
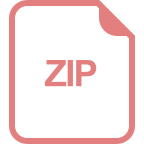
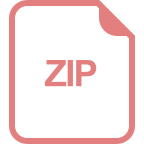
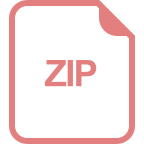
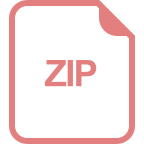